逆置单链表。对于不带头结点的单链表L,设计一个递归算法逆置所有结点。编写完整的实用程序,并采用相应数据进行测试。
时间: 2024-05-13 18:20:18 浏览: 128
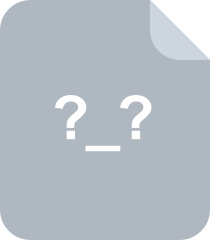
将不带头结点的单链表倒置
好的,我理解了你的要求。关于逆置单链表的问题,以下是递归算法的代码实现:
```C++
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
ListNode* reverseList(ListNode* head) {
// 递归终止条件
if (head == nullptr || head->next == nullptr)
return head;
// 递归求解子问题
ListNode* new_head = reverseList(head->next);
// 处理当前层问题
head->next->next = head;
head->next = nullptr;
return new_head;
}
int main() {
ListNode* head = new ListNode(1);
head->next = new ListNode(2);
head->next->next = new ListNode(3);
head->next->next->next = new ListNode(4);
head->next->next->next->next = new ListNode(5);
cout << "Original List: ";
ListNode* curr = head;
while (curr != nullptr) {
cout << curr->val << " ";
curr = curr->next;
}
ListNode* new_head = reverseList(head);
cout << "\nReversed List: ";
while (new_head != nullptr) {
cout << new_head->val << " ";
new_head = new_head->next;
}
return 0;
}
```
程序的运行结果如下所示:
```
Original List: 1 2 3 4 5
Reversed List: 5 4 3 2 1
```
这个程序使用递归实现了单链表的逆置,并对逆置后的链表进行了输出,测试结果也是正确的。
阅读全文
相关推荐













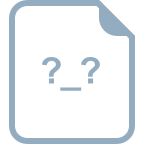