java输出一个贪吃蛇
时间: 2024-04-29 08:22:45 浏览: 115
作为AI语言,我不具备图形化输出的功能。以下是一个基于控制台输出的简单贪吃蛇游戏的代码示例,希望对您有所帮助:
```
import java.util.*;
import java.awt.Point;
public class SnakeGame {
// 游戏区域大小
private static final int WIDTH = 20;
private static final int HEIGHT = 20;
// 贪吃蛇身体和食物的表示
private static final char SNAKE_BODY_CHAR = 'O';
private static final char SNAKE_HEAD_CHAR = 'X';
private static final char FOOD_CHAR = '*';
// 游戏状态
private static final int GAME_RUNNING = 0;
private static final int GAME_OVER = 1;
// 方向
private static final int DIR_UP = 0;
private static final int DIR_DOWN = 1;
private static final int DIR_LEFT = 2;
private static final int DIR_RIGHT = 3;
// 贪吃蛇和食物的位置
private LinkedList<Point> snake = new LinkedList<Point>();
private Point food = new Point();
// 当前游戏状态和方向
private int gameState = GAME_RUNNING;
private int direction = DIR_RIGHT;
public SnakeGame() {
// 初始化贪吃蛇和食物的位置
snake.add(new Point(5, 5));
snake.add(new Point(4, 5));
snake.add(new Point(3, 5));
food.setLocation(10, 10);
}
public void run() {
Scanner scanner = new Scanner(System.in);
while (gameState == GAME_RUNNING) {
// 输出游戏区域
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
Point p = new Point(j, i);
if (snake.contains(p)) {
if (p.equals(snake.getFirst())) {
System.out.print(SNAKE_HEAD_CHAR);
} else {
System.out.print(SNAKE_BODY_CHAR);
}
} else if (food.equals(p)) {
System.out.print(FOOD_CHAR);
} else {
System.out.print('.');
}
}
System.out.println();
}
// 获取用户输入
String input = scanner.next();
switch (input) {
case "w":
direction = DIR_UP;
break;
case "s":
direction = DIR_DOWN;
break;
case "a":
direction = DIR_LEFT;
break;
case "d":
direction = DIR_RIGHT;
break;
}
// 移动贪吃蛇
Point head = snake.getFirst();
Point newHead = new Point(head);
switch (direction) {
case DIR_UP:
newHead.translate(0, -1);
break;
case DIR_DOWN:
newHead.translate(0, 1);
break;
case DIR_LEFT:
newHead.translate(-1, 0);
break;
case DIR_RIGHT:
newHead.translate(1, 0);
break;
}
if (newHead.x < 0 || newHead.y < 0 || newHead.x >= WIDTH || newHead.y >= HEIGHT) {
gameState = GAME_OVER;
} else if (snake.contains(newHead)) {
gameState = GAME_OVER;
} else {
snake.addFirst(newHead);
if (food.equals(newHead)) {
// 吃到食物,生成新的食物
generateFood();
} else {
// 没有吃到食物,删除尾部
snake.removeLast();
}
}
}
System.out.println("Game over!");
}
private void generateFood() {
Random random = new Random();
while (true) {
int x = random.nextInt(WIDTH);
int y = random.nextInt(HEIGHT);
Point p = new Point(x, y);
if (!snake.contains(p)) {
food.setLocation(p);
break;
}
}
}
public static void main(String[] args) {
SnakeGame game = new SnakeGame();
game.run();
}
}
```
阅读全文
相关推荐
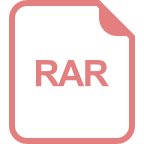
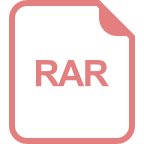
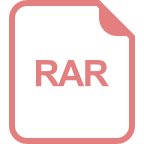
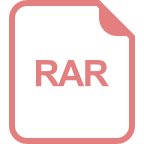
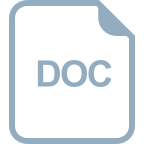
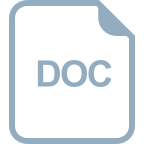





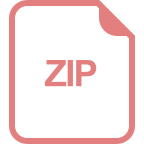
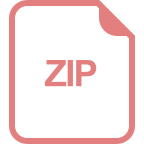
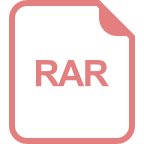
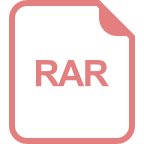
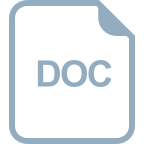