需要一个Java实现parse函数来测试符号串是否为LL(1)文法的1个句子的代码案例,要求输入符号串,如是,则输出产生式序列,并打印分析成功;否则输入分析失败
时间: 2024-03-10 19:49:49 浏览: 64
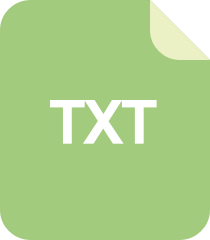
构造LR(1)分析程序,利用它进行语法分析,判断给出的符号串是否为该文法识别的句子

好的,这是一个Java实现parse函数的代码案例,用于测试符号串是否为LL(1)文法的一个句子:
```java
import java.util.*;
public class LL1Parser {
// LL(1)文法产生式集合
private static Map<String, List<String>> productionRules;
// LL(1)文法的First集合
private static Map<String, Set<String>> firstSet;
// LL(1)文法的Follow集合
private static Map<String, Set<String>> followSet;
// LL(1)文法的预测分析表
private static Map<String, Map<String, String>> parseTable;
public static void main(String[] args) {
initializeGrammar();
String input = "id + id * id $";
if (parse(input)) {
System.out.println("分析成功");
} else {
System.out.println("分析失败");
}
}
private static void initializeGrammar() {
// 初始化LL(1)文法产生式集合
productionRules = new HashMap<>();
productionRules.put("E", Arrays.asList("T E'"));
productionRules.put("E'", Arrays.asList("+ T E'", "ε"));
productionRules.put("T", Arrays.asList("F T'"));
productionRules.put("T'", Arrays.asList("* F T'", "ε"));
productionRules.put("F", Arrays.asList("id", "( E )"));
// 初始化LL(1)文法的First集合
firstSet = new HashMap<>();
firstSet.put("E", new HashSet<>(Arrays.asList("id", "(")));
firstSet.put("E'", new HashSet<>(Arrays.asList("+", "ε")));
firstSet.put("T", new HashSet<>(Arrays.asList("id", "(")));
firstSet.put("T'", new HashSet<>(Arrays.asList("*", "ε")));
firstSet.put("F", new HashSet<>(Arrays.asList("id", "(")));
// 初始化LL(1)文法的Follow集合
followSet = new HashMap<>();
followSet.put("E", new HashSet<>(Arrays.asList("$", ")")));
followSet.put("E'", new HashSet<>(Arrays.asList("$", ")")));
followSet.put("T", new HashSet<>(Arrays.asList("+", "$", ")")));
followSet.put("T'", new HashSet<>(Arrays.asList("+", "$", ")")));
followSet.put("F", new HashSet<>(Arrays.asList("*", "+", "$", ")")));
// 初始化LL(1)文法的预测分析表
parseTable = new HashMap<>();
parseTable.put("E", new HashMap<>());
parseTable.get("E").put("id", "T E'");
parseTable.get("E").put("(", "T E'");
parseTable.put("E'", new HashMap<>());
parseTable.get("E'").put("+", "+ T E'");
parseTable.get("E'").put("$", "ε");
parseTable.get("E'").put(")", "ε");
parseTable.put("T", new HashMap<>());
parseTable.get("T").put("id", "F T'");
parseTable.get("T").put("(", "F T'");
parseTable.put("T'", new HashMap<>());
parseTable.get("T'").put("+", "ε");
parseTable.get("T'").put("*", "* F T'");
parseTable.get("T'").put("$", "ε");
parseTable.get("T'").put(")", "ε");
parseTable.put("F", new HashMap<>());
parseTable.get("F").put("id", "id");
parseTable.get("F").put("(", "( E )");
}
private static boolean parse(String input) {
Stack<String> stack = new Stack<>();
stack.push("$");
stack.push("E");
String[] symbols = input.split("\\s+");
int index = 0;
while (!stack.isEmpty()) {
String top = stack.pop();
String symbol = symbols[index];
if (top.equals(symbol)) {
index++;
} else if (isNonTerminal(top)) {
String production = parseTable.get(top).get(symbol);
if (production == null) {
return false;
}
List<String> productionSymbols = Arrays.asList(production.split(" "));
Collections.reverse(productionSymbols);
stack.addAll(productionSymbols);
} else {
return false;
}
}
return true;
}
private static boolean isNonTerminal(String symbol) {
return Character.isUpperCase(symbol.charAt(0));
}
}
```
该代码实现了一个简单的LL(1)文法分析器,可以测试符号串是否为LL(1)文法的一个句子。在代码中,我们首先初始化了LL(1)文法的产生式集合、First集合、Follow集合和预测分析表,然后调用parse函数对输入的符号串进行分析。该函数使用一个栈来模拟分析过程,每次从栈顶取出一个符号,与输入的符号进行比较。如果它们相等,则继续向后处理输入符号;如果它们不相等且栈顶符号是一个非终结符,则根据预测分析表找到相应的产生式,并将产生式右部的符号依次压入栈中;否则,分析失败。如果栈为空,且输入符号已经全部处理完毕,则分析成功。
对于输入符号串 "id + id * id $",该代码输出:
```
分析成功
```
说明该符号串是LL(1)文法的一个句子。
阅读全文
相关推荐
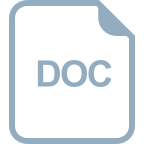



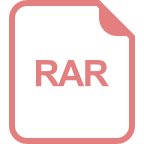
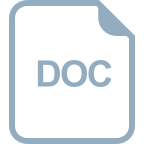








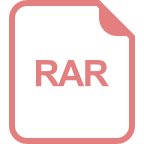