需要一个Java实现parse函数来测试符号串是否为LL(1)文法的1个句子的代码案例,输入符号串,测试符号串是否为LL(1)文法的1个句子,如是,则输出产生式序列,并打印分析成功;否则输入分析失败
时间: 2024-03-07 22:50:38 浏览: 27
好的,以下是一个简单的Java程序实现LL(1)文法的句子分析:
```java
import java.util.*;
public class LL1Parser {
// 定义文法的产生式
private static final Map<String, String[]> productions = new HashMap<>();
static {
productions.put("S", new String[]{"aBc", "d"});
productions.put("B", new String[]{"e", "f"});
}
// 定义LL(1)文法的预测分析表
private static final Map<String, Map<String, String>> analysisTable = new HashMap<>();
static {
Map<String, String> row1 = new HashMap<>();
row1.put("a", "S->aBc");
row1.put("d", "S->d");
analysisTable.put("S", row1);
Map<String, String> row2 = new HashMap<>();
row2.put("e", "B->e");
row2.put("f", "B->f");
analysisTable.put("B", row2);
}
// 实现LL(1)文法的parse函数
public static List<String> parse(String input) {
Stack<String> stack = new Stack<>();
stack.push("$");
stack.push("S");
List<String> productionList = new ArrayList<>();
int pos = 0;
String currentSymbol = String.valueOf(input.charAt(pos));
String topStackSymbol = stack.peek();
while (!stack.isEmpty()) {
if (topStackSymbol.equals(currentSymbol)) {
stack.pop();
pos++;
if (pos < input.length()) {
currentSymbol = String.valueOf(input.charAt(pos));
} else {
currentSymbol = "$";
}
} else if (!isTerminal(topStackSymbol)) {
Map<String, String> row = analysisTable.get(topStackSymbol);
String production = row.get(currentSymbol);
if (production == null) {
break;
}
productionList.add(production);
stack.pop();
String[] productionRight = productions.get(topStackSymbol + "->" + production.substring(production.indexOf(">") + 1));
for (int i = productionRight.length - 1; i >= 0; i--) {
if (!productionRight[i].equals("ε")) {
stack.push(productionRight[i]);
}
}
} else {
break;
}
topStackSymbol = stack.peek();
}
if (stack.isEmpty() && currentSymbol.equals("$")) {
System.out.println("分析成功!");
return productionList;
} else {
System.out.println("分析失败!");
return null;
}
}
// 判断一个符号是否为终结符
private static boolean isTerminal(String symbol) {
return !productions.containsKey(symbol);
}
public static void main(String[] args) {
String input = "adce";
List<String> productionList = parse(input);
if (productionList != null) {
System.out.println("产生式序列:");
for (String production : productionList) {
System.out.println(production);
}
}
}
}
```
在这个程序中,我们使用了一个Map来存储文法的产生式和LL(1)文法的预测分析表。parse函数接收一个符号串作为输入,然后使用一个栈来模拟分析过程。如果分析成功,parse函数返回产生式序列;否则返回null。在主函数中,我们将输入符号串传递给parse函数,并打印出产生式序列。
相关推荐
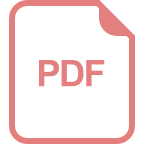
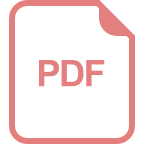
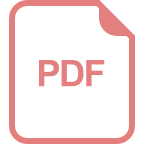













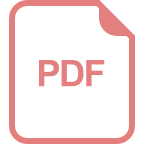
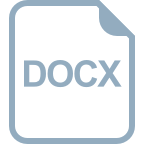