用java写这道题Given a 0-indexed 2D grid of size m x n, you should find the matrix answer of size m x n. The value of each cell (r, c) of the matrix answer is calculated in the following way: Let topLeft[r][c] be the number of distinct values in the top-left diagonal of the cell (r, c) in the matrix grid. Let bottomRight[r][c] be the number of distinct values in the bottom-right diagonal of the cell (r, c) in the matrix grid. Then answer[r][c] = |topLeft[r][c] - bottomRight[r][c]|. Return the matrix answer. A matrix diagonal is a diagonal line of cells starting from some cell in either the topmost row or leftmost column and going in the bottom-right direction until reaching the matrix's end. A cell (r1, c1) belongs to the top-left diagonal of the cell (r, c), if both belong to the same diagonal and r1 < r. Similarly is defined bottom-right diagonal.
时间: 2024-02-13 15:05:25 浏览: 26
以下是Java代码实现:
```
class Solution {
public int[][] diagonalDifference(int[][] grid) {
int m = grid.length;
int n = grid[0].length;
int[][] answer = new int[m][n];
for (int r = 0; r < m; r++) {
for (int c = 0; c < n; c++) {
Set<Integer> topLeft = new HashSet<>();
Set<Integer> bottomRight = new HashSet<>();
// Calculate top-left diagonal
for (int i = r-1, j = c-1; i >= 0 && j >= 0; i--, j--) {
topLeft.add(grid[i][j]);
}
// Calculate bottom-right diagonal
for (int i = r+1, j = c+1; i < m && j < n; i++, j++) {
bottomRight.add(grid[i][j]);
}
// Calculate answer
answer[r][c] = Math.abs(topLeft.size() - bottomRight.size());
}
}
return answer;
}
}
```
在这个解法中,我们首先定义一个大小为m x n的answer数组,并初始化为0。然后,我们遍历每个单元格(r, c)。对于每个单元格,我们使用两个嵌套循环计算它的top-left和bottom-right对角线中不同的值。我们使用两个HashSet来存储这些值,因为HashSet可以自动去重。最后,我们计算这个单元格的答案,并将其放入answer数组中。最后,我们返回answer数组。
相关推荐
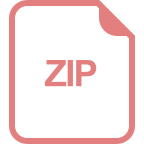
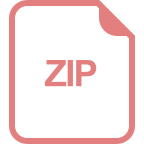














