model.add(LSTM(units=128, input_shape=(X_train.shape[-2:])))给这段代码加一个注意力机制
时间: 2024-06-09 09:06:38 浏览: 116
可以通过添加一个Attention层来实现注意力机制,代码如下:
```
from keras.layers import Layer, Input, Dense, LSTM, Attention, Concatenate
from keras.models import Model
# 定义Attention层
class AttentionLayer(Layer):
def __init__(self, **kwargs):
super(AttentionLayer, self).__init__(**kwargs)
def build(self, input_shape):
self.W = self.add_weight(name="att_weight", shape=(input_shape[-1], 1), initializer="normal")
self.b = self.add_weight(name="att_bias", shape=(input_shape[1], 1), initializer="zeros")
super(AttentionLayer, self).build(input_shape)
def call(self, x):
e = K.tanh(K.dot(x, self.W) + self.b)
a = K.softmax(e, axis=1)
output = x * a
return K.sum(output, axis=1)
def compute_output_shape(self, input_shape):
return (input_shape[0], input_shape[-1])
# 定义模型
inputs = Input(shape=(X_train.shape[-2], X_train.shape[-1]))
lstm = LSTM(units=128, return_sequences=True)(inputs)
attention = AttentionLayer()(lstm)
outputs = Dense(1, activation='sigmoid')(attention)
model = Model(inputs=inputs, outputs=outputs)
```
这里定义了一个Attention层,将LSTM的输出作为输入,然后计算每个时间步的权重,最后将加权后的输出进行求和得到Attention结果,最终通过Dense层输出。需要注意的是,Attention层的输入为三维张量,因此需要将LSTM的return_sequences参数设置为True。
在模型训练时,需要将模型的损失函数设置为binary_crossentropy,代码如下:
```
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
model.fit(X_train, y_train, epochs=10, batch_size=64, validation_data=(X_test, y_test))
```
阅读全文
相关推荐
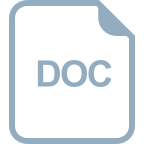
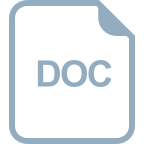
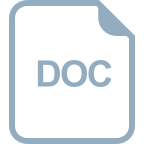












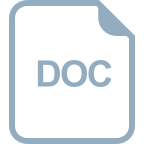
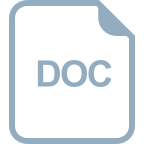
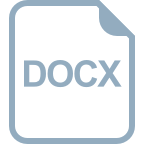