如何使用Pytorch计算矩阵乘法
时间: 2023-12-15 17:07:33 浏览: 36
在PyTorch中,可以使用`torch.matmul()`函数来进行矩阵乘法计算。该函数的输入可以是两个张量,也可以是多个张量。
下面是一个简单的例子,演示如何使用`torch.matmul()`函数计算两个矩阵的乘积:
```python
import torch
# 定义两个矩阵
a = torch.tensor([[1, 2], [3, 4]])
b = torch.tensor([[5, 6], [7, 8]])
# 计算矩阵乘积
c = torch.matmul(a, b)
# 输出结果
print(c)
```
运行以上代码,输出结果为:
```
tensor([[19, 22],
[43, 50]])
```
在实际使用中,也可以使用`@`运算符来进行矩阵乘法计算,例如:
```python
c = a @ b
```
上述代码和使用`torch.matmul()`函数计算矩阵乘积的效果是一样的。
相关问题
写一段Pytorch计算矩阵乘法的代码
可以使用 PyTorch 的 `matmul` 函数来实现矩阵乘法,示例代码如下:
``` python
import torch
# 定义两个矩阵
x = torch.randn(2, 3)
y = torch.randn(3, 4)
# 计算矩阵乘法
z = torch.matmul(x, y)
print(x)
print(y)
print(z)
```
其中,`torch.randn` 函数用于生成指定维度的随机数矩阵,`torch.matmul` 函数用于计算矩阵乘法。在这个示例中,先定义了两个矩阵 `x` 和 `y`,然后通过 `torch.matmul(x, y)` 计算它们的矩阵乘法结果,存储在变量 `z` 中,最后打印出来。
利用pytorch湖区矩阵乘法性能
PyTorch 是一个非常流行的深度学习框架,它提供了高效的张量操作。其中包括矩阵乘法,可以使用 PyTorch 中的 torch.mm() 或 torch.matmul() 函数来实现。
如果要利用 PyTorch 的矩阵乘法来提高性能,可以考虑以下几点:
1. 利用 GPU:PyTorch 可以利用 GPU 加速矩阵乘法,使用 CUDA 架构的 GPU 可以大大提高矩阵乘法的运算速度。
2. 使用批量矩阵乘法:如果有多个矩阵需要相乘,可以使用批量矩阵乘法,将它们一起进行计算,可以提高计算速度。
3. 优化矩阵形状:PyTorch 的矩阵乘法函数要求两个输入张量的维度满足一定的条件,可以通过改变矩阵的形状,使其适合矩阵乘法的要求,从而提高计算速度。
4. 使用半精度浮点数:PyTorch 支持使用半精度浮点数进行计算,这可以大大提高矩阵乘法的计算速度,但需要注意半精度浮点数会损失一定的精度。
总之,利用 PyTorch 的矩阵乘法可以提高性能,但需要根据具体情况选择合适的优化方法。
相关推荐
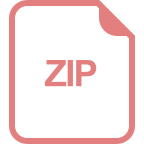
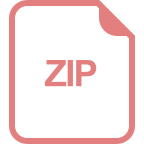
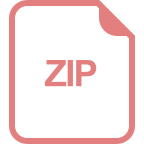












