C语言。编写一个程序,实现如下功能:包含一个生产者线程和一个消费者线程,它们有一个共享缓冲区;共享缓冲区是一个长度为10的字符型数组,该数组为全局变量;生产者从键盘获取一个用户输入的字符串(长度不超过255字符),然后将字符串逐字符地存入共享缓冲区;消费者从共享缓冲区中逐字符地读取并打印字符;当检测到输入字符串中的‘!”时,结束程序。
时间: 2024-02-16 08:01:34 浏览: 16
下面是一个基于 pthread 库的 C 语言程序,实现了上述功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define BUFFER_SIZE 10 // 缓冲区长度
#define INPUT_SIZE 256 // 输入字符串长度
char buffer[BUFFER_SIZE]; // 全局共享缓冲区
int in = 0, out = 0; // 缓冲区读写指针
pthread_mutex_t mutex; // 互斥锁
pthread_cond_t empty, full; // 条件变量
void *producer(void *arg) {
char input[INPUT_SIZE];
while (1) {
printf("Enter a string: ");
fgets(input, INPUT_SIZE, stdin);
for (int i = 0; input[i] != '\n' && input[i] != '\0'; i++) {
pthread_mutex_lock(&mutex);
while ((in + 1) % BUFFER_SIZE == out) // 缓冲区已满,等待
pthread_cond_wait(&empty, &mutex);
buffer[in] = input[i];
in = (in + 1) % BUFFER_SIZE;
pthread_cond_signal(&full);
pthread_mutex_unlock(&mutex);
if (input[i] == '!' && input[i+1] == '\0') { // 检测到输入字符串中的“!”
pthread_cond_signal(&full); // 唤醒消费者线程,让它检测到结束条件
return NULL;
}
}
}
}
void *consumer(void *arg) {
while (1) {
pthread_mutex_lock(&mutex);
while (in == out) // 缓冲区为空,等待
pthread_cond_wait(&full, &mutex);
putchar(buffer[out]);
out = (out + 1) % BUFFER_SIZE;
pthread_cond_signal(&empty);
pthread_mutex_unlock(&mutex);
if (buffer[out-1] == '!' && out == in) // 检测到结束条件
return NULL;
}
}
int main() {
pthread_t tid_producer, tid_consumer;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&empty, NULL);
pthread_cond_init(&full, NULL);
pthread_create(&tid_producer, NULL, producer, NULL);
pthread_create(&tid_consumer, NULL, consumer, NULL);
pthread_join(tid_producer, NULL);
pthread_join(tid_consumer, NULL);
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&empty);
pthread_cond_destroy(&full);
return 0;
}
```
程序中使用了互斥锁和条件变量来实现线程的同步和互斥。生产者线程从键盘获取输入字符串,逐字符地存入共享缓冲区;消费者线程从共享缓冲区中逐字符地读取并打印字符。当生产者线程检测到输入字符串中的“!”时,唤醒消费者线程,并结束程序。当消费者线程检测到结束条件时,结束程序。
相关推荐
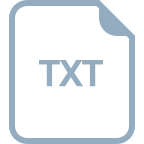
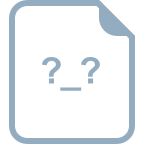
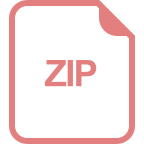














