c++扫雷详细步骤教程,使用easyx函数库,并附上完整代码
时间: 2023-12-14 07:39:23 浏览: 87
以下是使用EasyX函数库实现的c++扫雷完整代码,其中包括详细的注释说明:
```c++
#include <graphics.h>
#include <conio.h>
#include <time.h>
// 雷区的大小
const int ROWS = 20; // 行数
const int COLS = 20; // 列数
// 每个格子的大小
const int BLOCK_SIZE = 20;
// 雷的数量
const int MINE_NUM = 30;
// 雷的标志
const int MINE = -1;
// 游戏状态
const int PLAYING = 0; // 游戏进行中
const int WIN = 1; // 玩家胜利
const int LOSE = 2; // 玩家失败
// 雷区
int mines[ROWS][COLS];
// 点击状态
bool clicked[ROWS][COLS];
// 游戏状态
int gameState;
// 初始化雷区
void initMines()
{
// 随机种子
srand((unsigned)time(NULL));
// 先将雷区清空
memset(mines, 0, sizeof(mines));
// 在雷区随机放置雷
for (int i = 0; i < MINE_NUM; i++)
{
int row = rand() % ROWS;
int col = rand() % COLS;
if (mines[row][col] == MINE)
{
// 如果该位置已经有雷了,重新生成
i--;
}
else
{
// 放置雷
mines[row][col] = MINE;
}
}
}
// 绘制雷区
void drawMines()
{
// 绘制每个格子
for (int row = 0; row < ROWS; row++)
{
for (int col = 0; col < COLS; col++)
{
// 计算格子的位置
int x = col * BLOCK_SIZE;
int y = row * BLOCK_SIZE;
// 绘制格子的边框
rectangle(x, y, x + BLOCK_SIZE, y + BLOCK_SIZE);
// 如果该格子已经被点击
if (clicked[row][col])
{
// 如果是雷
if (mines[row][col] == MINE)
{
// 绘制红色的正方形
setfillcolor(RED);
solidrectangle(x + 2, y + 2, x + BLOCK_SIZE - 2, y + BLOCK_SIZE - 2);
}
else
{
// 绘制数字
int nearbyMines = 0;
// 计算周围雷的数量
for (int r = row - 1; r <= row + 1; r++)
{
for (int c = col - 1; c <= col + 1; c++)
{
if (r >= 0 && r < ROWS && c >= 0 && c < COLS && mines[r][c] == MINE)
{
nearbyMines++;
}
}
}
// 绘制数字
char str[2];
str[0] = '0' + nearbyMines;
str[1] = '\0';
outtextxy(x + 5, y + 5, str);
}
}
}
}
}
// 绘制游戏界面
void drawGame()
{
// 绘制雷区
drawMines();
// 刷新画面
flush();
}
// 初始化游戏
void initGame()
{
// 初始化游戏状态
gameState = PLAYING;
// 初始化雷区
initMines();
// 将所有格子的点击状态初始化为未点击
memset(clicked, false, sizeof(clicked));
// 绘制游戏界面
drawGame();
}
// 点击格子
void clickBlock(int row, int col)
{
// 如果该格子已经被点击,直接返回
if (clicked[row][col])
{
return;
}
// 标记该格子已经被点击
clicked[row][col] = true;
// 如果该格子是雷
if (mines[row][col] == MINE)
{
gameState = LOSE;
return;
}
// 计算周围雷的数量
int nearbyMines = 0;
for (int r = row - 1; r <= row + 1; r++)
{
for (int c = col - 1; c <= col + 1; c++)
{
if (r >= 0 && r < ROWS && c >= 0 && c < COLS && mines[r][c] == MINE)
{
nearbyMines++;
}
}
}
// 如果周围没有雷,递归点击周围的格子
if (nearbyMines == 0)
{
for (int r = row - 1; r <= row + 1; r++)
{
for (int c = col - 1; c <= col + 1; c++)
{
if (r >= 0 && r < ROWS && c >= 0 && c < COLS)
{
clickBlock(r, c);
}
}
}
}
// 判断是否胜利
int clickedBlocks = 0;
for (int r = 0; r < ROWS; r++)
{
for (int c = 0; c < COLS; c++)
{
if (clicked[r][c])
{
clickedBlocks++;
}
}
}
if (clickedBlocks == ROWS * COLS - MINE_NUM)
{
gameState = WIN;
}
}
int main()
{
// 初始化图形界面
initgraph(COLS * BLOCK_SIZE, ROWS * BLOCK_SIZE);
// 初始化游戏
initGame();
// 循环处理消息,直到游戏结束
while (gameState == PLAYING)
{
// 等待用户点击
MOUSEMSG mouse = GetMouseMsg();
if (mouse.uMsg == WM_LBUTTONDOWN)
{
// 计算点击的格子的坐标
int row = mouse.y / BLOCK_SIZE;
int col = mouse.x / BLOCK_SIZE;
// 点击该格子
clickBlock(row, col);
// 绘制游戏界面
drawGame();
}
}
// 游戏结束,显示结果
if (gameState == WIN)
{
outtextxy(100, 100, "You Win!");
}
else
{
outtextxy(100, 100, "You Lose!");
}
// 等待用户关闭窗口
getch();
// 关闭图形界面
closegraph();
return 0;
}
```
以上是使用EasyX函数库实现的c++扫雷完整代码,希望对你有所帮助。
相关推荐














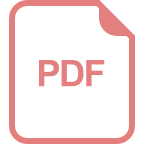