c++井字棋游戏代码easyx
时间: 2024-05-26 16:07:40 浏览: 25
C++井字棋游戏代码可以使用EasyX图形库进行实现,下面是一个简单的示例代码:
```
#include <graphics.h>
#include <conio.h>
const int kBoardSize = 3; // 棋盘大小
// 绘制棋盘
void DrawBoard() {
cleardevice(); // 清空屏幕
int width = getwidth() / kBoardSize;
int height = getheight() / kBoardSize;
// 绘制横线
for (int i = 1; i < kBoardSize; i++) {
line(0, i * height, getwidth(), i * height);
}
// 绘制竖线
for (int i = 1; i < kBoardSize; i++) {
line(i * width, 0, i * width, getheight());
}
}
// 下棋
void PlayChess(int row, int col, bool is_black) {
int x = col * getwidth() / kBoardSize + getwidth() / kBoardSize / 2;
int y = row * getheight() / kBoardSize + getheight() / kBoardSize / 2;
if (is_black) {
setcolor(BLACK);
circle(x, y, getwidth() / kBoardSize / 2 - 10);
} else {
setcolor(WHITE);
line(x - getwidth() / kBoardSize / 2 + 10, y - getheight() / kBoardSize / 2 + 10,
x + getwidth() / kBoardSize / 2 - 10, y + getheight() / kBoardSize / 2 - 10);
line(x - getwidth() / kBoardSize / 2 + 10, y + getheight() / kBoardSize / 2 - 10,
x + getwidth() / kBoardSize / 2 - 10, y - getheight() / kBoardSize / 2 + 10);
}
}
int main() {
initgraph(600, 600);
DrawBoard();
bool is_black = true; // 黑棋先手
while (true) {
if (_kbhit()) { // 如果有按键按下
char ch = _getch();
if (ch == 27) break; // 如果是Esc键,退出游戏
int row = -1;
int col = -1;
bool can_put = false;
switch (ch) {
case 'q': row = 0; col = 0; can_put = true; break;
case 'w': row = 0; col = 1; can_put = true; break;
case 'e': row = 0; col = 2; can_put = true; break;
case 'a': row = 1; col = 0; can_put = true; break;
case 's': row = 1; col = 1; can_put = true; break;
case 'd': row = 1; col = 2; can_put = true; break;
case 'z': row = 2; col = 0; can_put = true; break;
case 'x': row = 2; col = 1; can_put = true; break;
case 'c': row = 2; col = 2; can_put = true; break;
}
if (can_put) {
PlayChess(row, col, is_black);
is_black = !is_black;
}
}
}
closegraph();
return 0;
}
```
此示例中,通过`initgraph`函数初始化图形界面,然后在`DrawBoard`函数中绘制棋盘,`PlayChess`函数用于在棋盘上下棋。在`main`函数中,通过`_kbhit`函数检测键盘是否有按键按下,然后根据按键的不同来确定落子的位置,通过`PlayChess`函数在棋盘上下棋,并通过`is_black`变量来控制黑白棋的交替。当按下Esc键时,游戏退出。
相关推荐
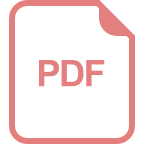
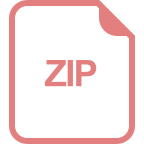
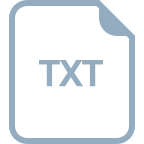














