.如果不用多线程完成图片复制并改名,代码如何编写?(不可以在复制时就改名,需要先同文件名复制进来,再改名) 2.如果采用多线程,但是不加锁,即一个线程完成复制,另一个完成改名,代码如何编写/执行结果是什么样? 3.采用多线程加锁方式,代码如何编写?
时间: 2023-09-24 07:11:23 浏览: 77
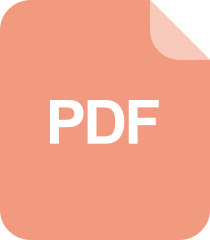
java多线程复制文件的实例代码
1. 不使用多线程的图片复制并改名代码示例:
```python
import shutil
import os
def copy_and_rename(src_dir, dst_dir):
for file in os.listdir(src_dir):
src_file = os.path.join(src_dir, file)
dst_file = os.path.join(dst_dir, file)
shutil.copy(src_file, dst_file)
new_name = file[:-4] + '_new' + file[-4:]
new_file = os.path.join(dst_dir, new_name)
os.rename(dst_file, new_file)
src_dir = 'path/to/source/directory'
dst_dir = 'path/to/destination/directory'
copy_and_rename(src_dir, dst_dir)
```
2. 如果采用多线程,但是不加锁,即一个线程完成复制,另一个完成改名,代码可能会出现意想不到的错误,因为改名的线程可能会尝试更改尚未完全复制的文件。代码示例如下:
```python
import shutil
import os
import threading
def copy_files(src_dir, dst_dir):
for file in os.listdir(src_dir):
src_file = os.path.join(src_dir, file)
dst_file = os.path.join(dst_dir, file)
shutil.copy(src_file, dst_file)
def rename_files(dst_dir):
for file in os.listdir(dst_dir):
if not file.endswith('_new'):
old_name = os.path.join(dst_dir, file)
new_name = os.path.join(dst_dir, file[:-4] + '_new' + file[-4:])
os.rename(old_name, new_name)
src_dir = 'path/to/source/directory'
dst_dir = 'path/to/destination/directory'
t1 = threading.Thread(target=copy_files, args=(src_dir, dst_dir))
t2 = threading.Thread(target=rename_files, args=(dst_dir,))
t1.start()
t2.start()
```
3. 使用多线程加锁方式的代码示例如下:
```python
import shutil
import os
import threading
def copy_and_rename(src_dir, dst_dir, lock):
for file in os.listdir(src_dir):
src_file = os.path.join(src_dir, file)
dst_file = os.path.join(dst_dir, file)
shutil.copy(src_file, dst_file)
new_name = file[:-4] + '_new' + file[-4:]
new_file = os.path.join(dst_dir, new_name)
lock.acquire()
os.rename(dst_file, new_file)
lock.release()
src_dir = 'path/to/source/directory'
dst_dir = 'path/to/destination/directory'
lock = threading.Lock()
t1 = threading.Thread(target=copy_and_rename, args=(src_dir, dst_dir, lock))
t2 = threading.Thread(target=copy_and_rename, args=(src_dir, dst_dir, lock))
t1.start()
t2.start()
t1.join()
t2.join()
```
在这个代码示例中,我们使用了 `threading.Lock()` 对象来确保在更改文件名时只有一个线程能够访问目标文件夹。每个线程都调用 `lock.acquire()` 来获取锁,并在完成任务后调用 `lock.release()` 释放锁。这样就可以避免两个线程同时尝试更改同一个文件的可能性,并且代码可以安全地并行运行。
阅读全文
相关推荐
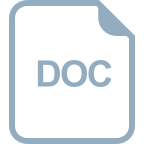
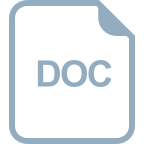
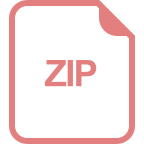
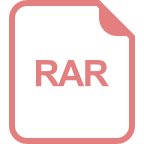
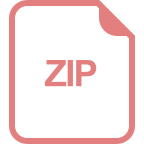
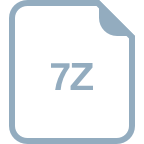
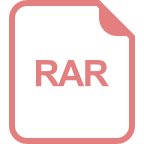
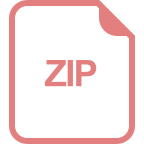
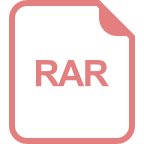
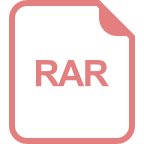
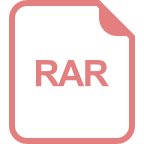
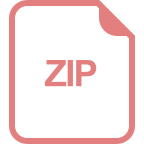
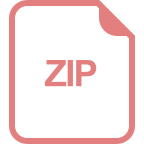
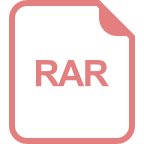
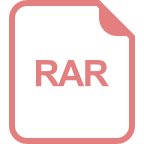


