原始图转化成对偶图的过程代码
时间: 2023-10-22 20:04:54 浏览: 27
对于一个二维平面上的原始图,其对偶图可以通过以下步骤生成:
1. 对于每条边,在其中垂线上取一个点作为新的顶点。
2. 对于每个面,以它的重心为新的顶点。
3. 连接相邻的新顶点。
以下是Python实现代码:
```python
import numpy as np
def dual_graph(coord, edges):
"""
将原始图的坐标和边信息转化为对偶图的坐标和边信息
:param coord: 原始图中每个顶点的坐标,二维数组,每行表示一个顶点的坐标
:param edges: 原始图中边的信息,二维数组,每行表示一条边,两个元素是该边连接的两个顶点的索引
:return: 对偶图中每个顶点的坐标和边的信息,二维数组,每行表示一个顶点的坐标或一条边的两个端点的索引
"""
# 计算每个面的重心
faces = []
for e in edges:
x1, y1 = coord[e[0]]
x2, y2 = coord[e[1]]
center_x, center_y = (x1 + x2) / 2, (y1 + y2) / 2
faces.append((center_x, center_y))
faces = np.array(faces)
# 计算每条边中垂线上的点
new_vertices = []
for e in edges:
x1, y1 = coord[e[0]]
x2, y2 = coord[e[1]]
center_x, center_y = (x1 + x2) / 2, (y1 + y2) / 2
dx, dy = y2 - y1, x1 - x2
length = np.sqrt(dx * dx + dy * dy)
dx /= length
dy /= length
new_x, new_y = center_x + dy, center_y + dx
new_vertices.append((new_x, new_y))
new_vertices = np.array(new_vertices)
# 连接相邻的新顶点
new_edges = []
num_vertices = len(coord)
for i in range(num_vertices):
# 找到与该顶点相邻的所有边
neighbor_edges = []
for j in range(len(edges)):
if i in edges[j]:
neighbor_edges.append(j)
# 连接相邻的新顶点
for j in range(len(neighbor_edges)):
for k in range(j + 1, len(neighbor_edges)):
e1, e2 = neighbor_edges[j], neighbor_edges[k]
v1, v2 = e1 + num_vertices, e2 + num_vertices
new_edges.append((v1, v2))
# 返回新的坐标和边信息
vertices = np.concatenate((coord, faces, new_vertices), axis=0)
edges = np.array(new_edges)
return vertices, edges
```
其中,`coord`是一个二维数组,每行表示原始图中一个顶点的坐标;`edges`是一个二维数组,每行表示原始图中一条边的两个顶点的索引。函数返回一个二维数组,其中每行表示对偶图中一个顶点的坐标或一条边的两个端点的索引。
相关推荐
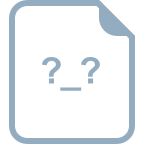








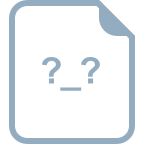
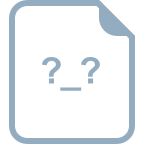
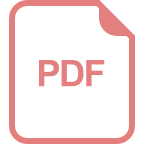
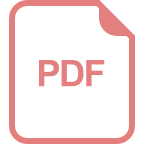
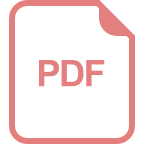
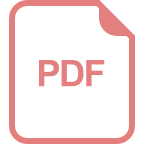
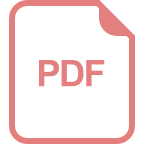