predict = model.predict(test_x) plt.plot(predict, label='predict') plt.plot(test_y, label='ground true') plt.legend() plt.show()
时间: 2023-09-28 22:06:18 浏览: 90
这段代码是用来绘制预测值和真实值的曲线图。首先,`model.predict(test_x)`使用模型对测试数据进行预测,得到预测值。然后,通过调用`plt.plot()`函数将预测值和真实值分别绘制在同一张图上。`label='predict'`表示预测值的标签,`label='ground true'`表示真实值的标签。再次调用`plt.legend()`函数添加图例,并调用`plt.show()`函数显示图像。这样可以比较预测值和真实值之间的差异。
相关问题
train_predict = model.predict(X_train) test_predict = model.predict(X_test) train_predict = scaler.inverse_transform(train_predict) y_train = scaler.inverse_transform([y_train]) test_predict = scaler.inverse_transform(test_predict) y_test = scaler.inverse_transform([y_test]) plt.plot(y_train, label='train') plt.plot(train_predict, label='train predict') plt.plot(y_test, label='test') plt.plot(test_predict, label='test predict') plt.legend() plt.show()报错Traceback (most recent call last): File "C:\Users\马斌\Desktop\cnn测试\cnn改.py", line 48, in <module> train_predict = scaler.inverse_transform(train_predict) File "D:\python\python3.9.5\pythonProject\venv\lib\site-packages\sklearn\preprocessing\_data.py", line 541, in inverse_transform X -= self.min_ ValueError: non-broadcastable output operand with shape (110,1) doesn't match the broadcast shape (110,4)
这个错误通常是由于数组形状不兼容而导致的。在您的代码中,似乎您正在尝试将形状为(110,1)的数组与形状为(110,4)的数组进行广播操作,从而导致此错误。
您可以尝试使用 `reshape()` 方法来重新调整数组的形状,以确保它们具有兼容的形状。例如,如果您想将形状为(110,1)的数组转换为形状为(110,4)的数组,可以使用以下代码:
```python
train_predict = np.tile(train_predict, (1, 4))
```
这将使用 `tile()` 函数将 `train_predict` 数组沿列方向复制 4 次,以便它的形状变为(110,4)。然后,您可以再次尝试执行广播操作。
# 加载数据 X = np.load("X_od.npy") Y = np.load("Y_od.npy") # 数据归一化 max = np.max(X) X = X / max Y = Y / max # 划分训练集、验证集、测试集 train_x = X[:1000] train_y = Y[:1000] val_x = X[1000:1150] val_y = Y[1000:1150] test_x = X[1150:] test_y = Y # 构建LSTM模型 model = Sequential() model.add(LSTM(units=64, input_shape=(5, 109),return_sequences=True)) model.add(Dropout(0.2)) model.add(Dense(units=1, activation='linear')) # 原为Dense(units=109) model.summary() # 编译模型 model.compile(optimizer='adam', loss='mse') # 训练模型 history = model.fit(train_x, train_y, epochs=50, batch_size=32, validation_data=(val_x, val_y), verbose=1, shuffle=False) # 评估模型 test_loss = model.evaluate(test_x, test_y) print('Test loss:', test_loss) # 模型预测 train_predict = model.predict(train_x) val_predict = model.predict(val_x) test_predict = model.predict(test_x) # 预测结果可视化 plt.figure(figsize=(20, 8)) plt.plot(train_y[-100:], label='true') plt.plot(train_predict[-100:], label='predict') plt.legend() plt.title('Training set') plt.show() plt.figure(figsize=(20, 8)) plt.plot(val_y[-50:], label='true') plt.plot(val_predict[-50:], label='predict') plt.legend() plt.title('Validation set') plt.show() plt.figure(figsize=(20, 8)) plt.plot(test_y[:50], label='true') plt.plot(test_predict[:50], label='predict') plt.legend() plt.title('Test set') plt.show()如何修改这段代码的数据维度让其可正常运行
根据代码,输入数据的维度为 (样本数, 时间步长, 特征数),其中,样本数为 1000,时间步长为 5,特征数为 109。因此,如果要修改数据维度,需要将输入数据的形状调整为 (样本数, 时间步长, 新的特征数)。
如果你想将特征数从 109 调整为其他值,可以使用特征选择或降维等技术来减少特征数量,也可以直接将特征数修改为新的值。如果你想将特征数修改为一个更小的值,例如 64,可以修改以下代码:
```
X = np.load("X_od.npy")
Y = np.load("Y_od.npy")
# 数据归一化
max = np.max(X)
X = X / max
Y = Y / max
# 将特征数从 109 修改为 64
X = X[:, :, :64]
# 划分训练集、验证集、测试集
train_x = X[:1000]
train_y = Y[:1000]
val_x = X[1000:1150]
val_y = Y[1000:1150]
test_x = X[1150:]
test_y = Y
# 构建LSTM模型
model = Sequential()
model.add(LSTM(units=64, input_shape=(5, 64), return_sequences=True))
model.add(Dropout(0.2))
model.add(Dense(units=1, activation='linear'))
model.summary()
# 编译模型
model.compile(optimizer='adam', loss='mse')
# 训练模型
history = model.fit(train_x, train_y, epochs=50, batch_size=32, validation_data=(val_x, val_y), verbose=1, shuffle=False)
# 评估模型
test_loss = model.evaluate(test_x, test_y)
print('Test loss:', test_loss)
# 模型预测
train_predict = model.predict(train_x)
val_predict = model.predict(val_x)
test_predict = model.predict(test_x)
# 预测结果可视化
plt.figure(figsize=(20, 8))
plt.plot(train_y[-100:], label='true')
plt.plot(train_predict[-100:], label='predict')
plt.legend()
plt.title('Training set')
plt.show()
plt.figure(figsize=(20, 8))
plt.plot(val_y[-50:], label='true')
plt.plot(val_predict[-50:], label='predict')
plt.legend()
plt.title('Validation set')
plt.show()
plt.figure(figsize=(20, 8))
plt.plot(test_y[:50], label='true')
plt.plot(test_predict[:50], label='predict')
plt.legend()
plt.title('Test set')
plt.show()
```
这里将特征数从 109 修改为 64,可以通过将 `X` 的最后一维切片来实现。同时,将模型中的 `input_shape` 修改为 `(5, 64)`。
阅读全文
相关推荐
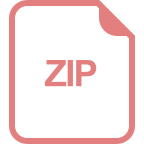
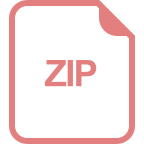













