给出若干个二维向量,如何用python对这些向量以顺时针排序?
时间: 2023-05-24 10:04:27 浏览: 107
可以通过向量的极角来进行排序,即将每个向量转换成极坐标形式,然后按极角从小到大排序。具体步骤如下:
1. 计算每个向量的极角,可以使用 `math.atan2(y, x)` 函数来计算,其中 `x` 和 `y` 分别为向量的横纵坐标。
2. 将每个向量及其极角组成一个元组,并将所有元组组成的列表进行排序,可以使用 `sorted()` 函数。
3. 对排序后的元组列表,只取出每个元组中的向量,即可得到按顺时针排序后的向量列表。
以下是完整的代码示例:
```python
import math
def clockwise_sort(vectors):
angles = [(v, math.atan2(v[1], v[0])) for v in vectors]
sorted_angles = sorted(angles, key=lambda x: x[1])
sorted_vectors = [a[0] for a in sorted_angles]
return sorted_vectors
```
其中 `vectors` 是要排序的向量列表。
示例:
```python
vectors = [(1, 0), (0, 1), (-1, 0), (0, -1)]
sorted_vectors = clockwise_sort(vectors)
print(sorted_vectors) # 输出 [(-1, 0), (0, 1), (1, 0), (0, -1)]
```
可以看到,经过排序后,向量 `(1, 0)` 和向量 `(0, 1)` 的顺序被调换了。
相关推荐
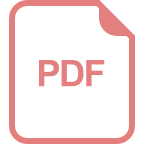
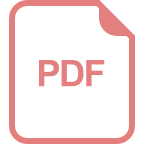














