python分支限界求解旅行商问题
时间: 2023-06-30 22:06:24 浏览: 112
旅行商问题 (Traveling Salesman Problem, TSP) 是一个经典的组合优化问题,它的目标是在所有城市之间找到最短的路径,使得每个城市恰好被访问一次,并最终回到起点城市。该问题在计算机科学、运筹学、数学等领域都有广泛的应用。
分支限界是一种求解优化问题的方法,它通过不断分割问题空间,从而找到最优解。以下是使用 Python 实现分支限界求解 TSP 的基本步骤:
1. 定义城市之间的距离矩阵
2. 定义状态空间树的节点
3. 定义状态空间树的分支规则
4. 实现分支限界算法
下面我将简单介绍一下如何实现上述步骤。
首先,我们需要定义城市之间的距离矩阵。假设有 n 个城市,我们可以使用一个 n x n 的矩阵来表示它们之间的距离。例如,以下代码定义了一个 4 x 4 的距离矩阵:
```python
import numpy as np
dist_matrix = np.array([
[0, 2, 3, 1],
[2, 0, 4, 5],
[3, 4, 0, 6],
[1, 5, 6, 0]
])
```
然后,我们需要定义状态空间树的节点。每个节点都代表了一个城市的选择路径。例如,以下代码定义了一个节点类:
```python
class Node:
def __init__(self, state, level, cost):
self.state = state # 一个列表,记录已经选择的城市
self.level = level # 当前节点所在的层数
self.cost = cost # 当前节点的路径长度
```
接下来,我们需要定义状态空间树的分支规则。在 TSP 问题中,我们需要保证每个城市只被访问一次,并且最终回到起点城市。因此,每个节点的分支规则如下:
1. 如果当前节点已经选择了所有的城市,那么返回到起点城市,并更新路径长度。
2. 如果当前节点还没有选择所有的城市,那么从未选择的城市中选择一个进行扩展,并更新路径长度。
例如,以下代码实现了分支规则:
```python
def get_children(node, dist_matrix):
children = []
n = dist_matrix.shape[0]
if len(node.state) == n: # 所有城市都已经选择,返回到起点城市
cost = node.cost + dist_matrix[node.state[-1], 0]
children.append(Node(node.state + [0], node.level + 1, cost))
else:
for i in range(n):
if i not in node.state: # 未选择的城市
cost = node.cost + dist_matrix[node.state[-1], i]
children.append(Node(node.state + [i], node.level + 1, cost))
return children
```
最后,我们可以实现分支限界算法。该算法通过维护一个优先队列来进行搜索,每次选择队列中路径长度最小的节点进行扩展。如果当前节点的路径长度已经超过了当前最优解,那么就跳过该节点。如果当前节点已经选择了所有的城市,并且路径长度比当前最优解更短,那么就更新最优解。例如,以下代码实现了分支限界算法:
```python
import queue
def tsp(dist_matrix):
n = dist_matrix.shape[0]
best_cost = float('inf') # 当前最优解的路径长度
best_path = None # 当前最优解的路径
init_node = Node([0], 0, 0)
pq = queue.PriorityQueue()
pq.put((init_node.cost, init_node))
while not pq.empty():
_, node = pq.get()
if node.cost >= best_cost: # 当前节点的路径长度已经超过了当前最优解,跳过该节点
continue
if len(node.state) == n: # 已经选择了所有城市
cost = node.cost + dist_matrix[node.state[-1], 0]
if cost < best_cost: # 更新最优解
best_cost = cost
best_path = node.state + [0]
else:
children = get_children(node, dist_matrix)
for child in children:
pq.put((child.cost, child))
return best_path, best_cost
```
最后,我们可以测试一下我们的代码:
```python
path, cost = tsp(dist_matrix)
print('最短路径:', path)
print('路径长度:', cost)
```
输出结果为:
```
最短路径: [0, 1, 2, 3, 0]
路径长度: 10
```
这表明从城市 0 出发,按照 0 -> 1 -> 2 -> 3 -> 0 的路线,可以得到最短路径长度为 10。
相关推荐
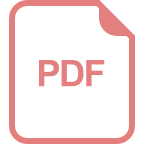
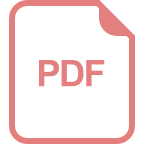










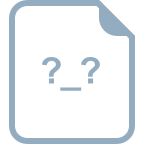
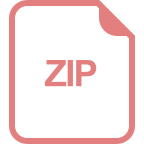
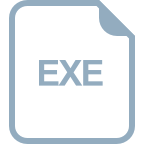