macchina.io使用C++解析JSON格式,给个复杂的示例
时间: 2024-03-15 18:45:50 浏览: 25
好的,以下是一个复杂的示例:
假设我们有以下JSON数据:
```json
{
"name": "John Doe",
"age": 30,
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA",
"zip": "12345"
},
"phoneNumbers": [
{
"type": "home",
"number": "555-1234"
},
{
"type": "work",
"number": "555-5678"
}
]
}
```
我们可以使用macchina.io中的JSON解析器来解析这个JSON数据,示例代码如下:
```c++
#include <iostream>
#include <vector>
#include <Poco/JSON/Parser.h>
#include <Poco/Dynamic/Var.h>
int main() {
std::string jsonString = R"({
"name": "John Doe",
"age": 30,
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA",
"zip": "12345"
},
"phoneNumbers": [
{
"type": "home",
"number": "555-1234"
},
{
"type": "work",
"number": "555-5678"
}
]
})";
Poco::JSON::Parser parser;
Poco::Dynamic::Var result = parser.parse(jsonString);
Poco::JSON::Object::Ptr root = result.extract<Poco::JSON::Object::Ptr>();
std::string name = root->getValue<std::string>("name");
int age = root->getValue<int>("age");
Poco::JSON::Object::Ptr address = root->getObject("address");
std::string street = address->getValue<std::string>("street");
std::string city = address->getValue<std::string>("city");
std::string state = address->getValue<std::string>("state");
std::string zip = address->getValue<std::string>("zip");
Poco::JSON::Array::Ptr phoneNumbers = root->getArray("phoneNumbers");
std::vector<std::pair<std::string, std::string>> phoneNumbersVec;
for (auto& item : *phoneNumbers) {
Poco::JSON::Object::Ptr phoneNumber = item.extract<Poco::JSON::Object::Ptr>();
std::string type = phoneNumber->getValue<std::string>("type");
std::string number = phoneNumber->getValue<std::string>("number");
phoneNumbersVec.push_back(std::make_pair(type, number));
}
std::cout << "name: " << name << std::endl;
std::cout << "age: " << age << std::endl;
std::cout << "street: " << street << std::endl;
std::cout << "city: " << city << std::endl;
std::cout << "state: " << state << std::endl;
std::cout << "zip: " << zip << std::endl;
for (auto& item : phoneNumbersVec) {
std::cout << "type: " << item.first << ", number: " << item.second << std::endl;
}
return 0;
}
```
这个示例中,我们首先定义了一个JSON字符串,然后使用Poco库中的JSON解析器来解析JSON数据。我们从根对象中获取了name和age字段,从address对象中获取了street、city、state和zip字段,从phoneNumbers数组中获取了所有的电话号码。最后,我们将获取到的字段打印出来。
相关推荐
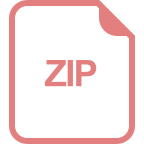
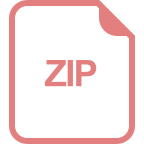














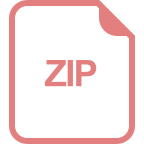