用c++第一题:定义一个满足如下要求的Date 类 (1)用下面的格式输出日期: 日/月/年 (2)可运行在日期上加一天操作; (3)设置日期。第二题:创建一个Employee类,该类中有字符数组,表示姓名、街道地址、市、省和邮政编码 把表示构造函数、ChangeNamne()、Display()的函数原型放在类定义中,构造函数初始化每个成员,Display()函数把完整的对象数据打印出来。其中的数据成员是保护的,函数是公共的
时间: 2024-02-26 16:59:21 浏览: 121
第一题:
```c++
#include <iostream>
using namespace std;
class Date {
private:
int day, month, year;
bool isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
int getDaysInMonth(int year, int month) {
int daysInMonth[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
if (month == 2 && isLeapYear(year)) {
return 29;
}
return daysInMonth[month - 1];
}
public:
Date(int day, int month, int year) {
this->day = day;
this->month = month;
this->year = year;
}
void display() {
cout << day << "/" << month << "/" << year << endl;
}
void addOneDay() {
int daysInMonth = getDaysInMonth(year, month);
if (day < daysInMonth) {
day++;
} else {
day = 1;
if (month < 12) {
month++;
} else {
month = 1;
year++;
}
}
}
void setDate(int day, int month, int year) {
this->day = day;
this->month = month;
this->year = year;
}
};
int main() {
Date date(31, 12, 2021);
date.display();
date.addOneDay();
date.display();
date.setDate(1, 1, 2022);
date.display();
return 0;
}
```
第二题:
```c++
#include <iostream>
using namespace std;
class Employee {
protected:
char name[50];
char streetAddress[100];
char city[50];
char province[50];
char postalCode[7];
public:
Employee(char name[], char streetAddress[], char city[], char province[], char postalCode[]) {
strcpy(this->name, name);
strcpy(this->streetAddress, streetAddress);
strcpy(this->city, city);
strcpy(this->province, province);
strcpy(this->postalCode, postalCode);
}
void changeName(char name[]) {
strcpy(this->name, name);
}
void display() {
cout << "Name: " << name << endl;
cout << "Street Address: " << streetAddress << endl;
cout << "City: " << city << endl;
cout << "Province: " << province << endl;
cout << "Postal Code: " << postalCode << endl;
}
};
int main() {
Employee employee("John Smith", "123 Main St", "Toronto", "ON", "M1H 1A1");
employee.display();
employee.changeName("Jane Doe");
employee.display();
return 0;
}
```
阅读全文
相关推荐
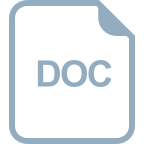
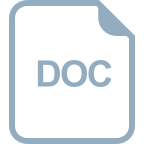
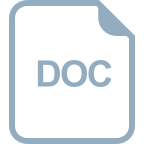


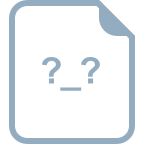
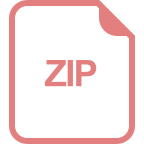











