仅设尾指针的循环队列java
时间: 2024-10-11 17:15:14 浏览: 43
在 Java 中,使用尾指针实现循环队列(Circular Queue)是一种空间效率较高的方法,因为它避免了对额外元素的引用。传统的队列需要两个指针,一个指向队首(front),另一个指向队尾(rear)。但在循环队列中,当 rear 指针到达队列末尾时,并不会变成 null,而是会重新指向队列的开头,形成一个环。
下面是一个简单的循环队列实现示例:
```java
public class CircularQueue {
private int[] queue;
private int front; // 队首索引
private int rear; // 尾部索引
private int capacity;
public CircularQueue(int size) {
this.capacity = size;
queue = new int[capacity];
front = -1;
rear = -1;
}
// 入队操作
public boolean enQueue(int value) {
if ((rear + 1) % capacity == front) { // 队满
return false;
}
rear = (rear + 1) % capacity; // 更新尾部索引并入队
queue[rear] = value;
if (front == -1) { // 如果队列为空,更新队首
front = rear;
}
return true;
}
// 出队操作
public int deQueue() {
if (front == -1) { // 队空
return -1; // 或者抛出异常
}
int removedValue = queue[front]; // 获取并移除队首元素
if (front == rear) { // 当前只有一个元素
front = rear = -1;
} else {
front = (front + 1) % capacity; // 更新队首
}
return removedValue;
}
// 判断队列是否为空
public boolean isEmpty() {
return front == -1;
}
// 判断队列是否已满
public boolean isFull() {
return (rear + 1) % capacity == front;
}
}
```
阅读全文
相关推荐
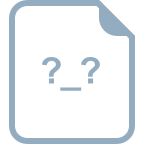
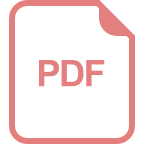
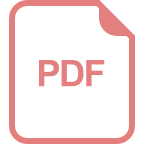


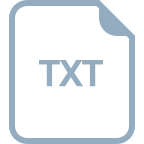
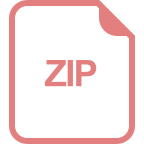
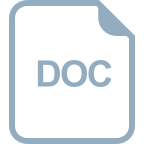
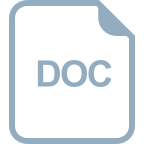









