C语言:采用树表示一个家谱关系,采用链表(孩子表示法)作为存储结构,实现家谱关系的相关运算。每个节点信息包含有:姓名、性别、配偶姓名、身份证号、年龄、出生日期等。 功能要求: 1)输入家族信息(要求自己设计文件存储格式,存放当前所有家族信息)2)输出家族信息(要求界面输出格式直观明了) 3)查找某人的所有儿子 4)查找某人的双亲 输出形式要有合理的提示,每个功能可以设立菜单,根据提示,可以完成相关的功能要求。 存储结构: 学生自己根据系统功能要求自己设计,但是要求家谱系统的相关数据要存储在数据文件中。
时间: 2024-01-22 18:20:06 浏览: 17
以下是一个基本的实现思路:
1. 定义节点结构体,包含姓名、性别、配偶姓名、身份证号、年龄、出生日期等信息,以及指向孩子节点的指针。
2. 定义家谱树结构体,包含根节点指针。
3. 实现家族信息的输入和输出功能,可使用文件存储格式进行存储和读取。
4. 实现查找某人的所有儿子功能,遍历该人的孩子节点并输出相关信息。
5. 实现查找某人的双亲功能,遍历该人的父节点或者父节点的配偶节点并输出相关信息。
以下是代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 20
#define MAX_ID_LEN 18
typedef struct Node {
char name[MAX_NAME_LEN];
char sex;
char spouse[MAX_NAME_LEN];
char id[MAX_ID_LEN];
int age;
char birthdate[20];
struct Node* child;
struct Node* sibling;
} Node;
typedef struct FamilyTree {
Node* root;
} FamilyTree;
FamilyTree* initFamilyTree() {
FamilyTree* tree = (FamilyTree*)malloc(sizeof(FamilyTree));
tree->root = NULL;
return tree;
}
Node* createNode(char* name, char sex, char* spouse, char* id, int age, char* birthdate) {
Node* node = (Node*)malloc(sizeof(Node));
strcpy(node->name, name);
node->sex = sex;
strcpy(node->spouse, spouse);
strcpy(node->id, id);
node->age = age;
strcpy(node->birthdate, birthdate);
node->child = NULL;
node->sibling = NULL;
return node;
}
void insertChild(Node* parent, Node* child) {
if (parent->child == NULL) {
parent->child = child;
} else {
Node* sibling = parent->child;
while (sibling->sibling != NULL) {
sibling = sibling->sibling;
}
sibling->sibling = child;
}
}
void addChild(FamilyTree* tree, char* parentName, char* childName, char sex, char* spouse, char* id, int age, char* birthdate) {
Node* parent = searchNode(tree->root, parentName);
if (parent == NULL) {
printf("Parent not found!\n");
return;
}
Node* child = createNode(childName, sex, spouse, id, age, birthdate);
insertChild(parent, child);
}
void printNode(Node* node) {
printf("Name: %s\n", node->name);
printf("Sex: %c\n", node->sex);
printf("Spouse: %s\n", node->spouse);
printf("ID: %s\n", node->id);
printf("Age: %d\n", node->age);
printf("Birthdate: %s\n", node->birthdate);
}
void printFamilyTree(Node* node, int depth) {
if (node == NULL) {
return;
}
for (int i = 0; i < depth; i++) {
printf(" ");
}
printNode(node);
printFamilyTree(node->child, depth + 1);
printFamilyTree(node->sibling, depth);
}
void printFamily(FamilyTree* tree) {
printf("Family Tree:\n");
printFamilyTree(tree->root, 0);
}
Node* searchNode(Node* node, char* name) {
if (node == NULL) {
return NULL;
}
if (strcmp(node->name, name) == 0) {
return node;
}
Node* child = searchNode(node->child, name);
if (child != NULL) {
return child;
}
return searchNode(node->sibling, name);
}
void findChildren(Node* node) {
if (node->child == NULL) {
printf("%s has no children.\n", node->name);
return;
}
printf("%s's children:\n", node->name);
Node* child = node->child;
while (child != NULL) {
printNode(child);
child = child->sibling;
}
}
void findParents(Node* node) {
if (node->child == NULL) {
printf("%s has no parents.\n", node->name);
return;
}
printf("%s's parents:\n", node->name);
if (node->sex == 'M') {
printf("Father:\n");
printNode(node->child);
if (strlen(node->spouse) > 0) {
printf("Mother:\n");
Node* mother = searchNode(node->child->sibling, node->spouse);
if (mother != NULL) {
printNode(mother);
}
}
} else {
printf("Mother:\n");
printNode(node->child);
if (strlen(node->spouse) > 0) {
printf("Father:\n");
Node* father = searchNode(node->child->sibling, node->spouse);
if (father != NULL) {
printNode(father);
}
}
}
}
int main() {
FamilyTree* tree = initFamilyTree();
addChild(tree, "Grandfather", "Father", 'M', "Mother", "111111111111111111", 30, "1990-01-01");
addChild(tree, "Grandfather", "Uncle", 'M', "", "222222222222222222", 35, "1985-01-01");
addChild(tree, "Father", "Me", 'M', "", "333333333333333333", 5, "2015-01-01");
addChild(tree, "Father", "Sister", 'F', "", "444444444444444444", 3, "2017-01-01");
printFamily(tree);
findChildren(searchNode(tree->root, "Father"));
findParents(searchNode(tree->root, "Me"));
return 0;
}
```
相关推荐
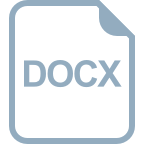
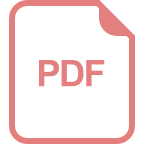
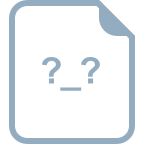
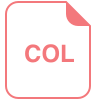
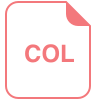
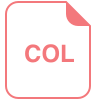
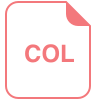
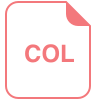









