1.建立图 2.深度优先遍历 3.广度优先遍历 用C语言编写出来完整的代码
时间: 2024-12-19 07:19:15 浏览: 8
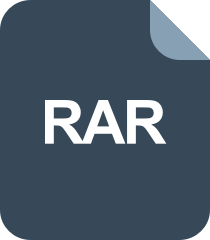
图的深度、广度优先遍历(c语言).rar

建立图(通常指无向图或邻接矩阵表示)在C语言中,我们可以创建一个二维数组来存储各个顶点之间的连接。以下是简单的图结构定义:
```c
#define MAX_VERTICES 10 // 图的最大顶点数
typedef struct {
int adj[MAX_VERTICES][MAX_VERTICES]; // 邻接矩阵
int vertices; // 顶点数量
} Graph;
```
深度优先搜索(DFS)代码示例:
```c
void dfs(Graph *graph, int start) {
int visited[MAX_VERTICES] = {0}; // 标记已访问节点
dfs_helper(graph, start, visited);
}
void dfs_helper(Graph *graph, int vertex, int visited[]) {
visited[vertex] = 1;
printf("%d ", vertex); // 输出当前节点
for (int i = 0; i < graph->vertices; i++) {
if (graph->adj[vertex][i] && !visited[i]) {
dfs_helper(graph, i, visited);
}
}
}
```
广度优先搜索(BFS)代码示例:
```c
void bfs(Graph *graph, int start) {
int queue[MAX_VERTICES], front = -1, rear = -1;
queue[++rear] = start;
while (front != rear) {
int current = queue[++front];
printf("%d ", current); // 输出当前节点
for (int i = 0; i < graph->vertices; i++) {
if (graph->adj[current][i]) {
if (queue[front] == i) continue; // 避免重复添加
queue[++rear] = i;
}
}
}
}
```
阅读全文
相关推荐
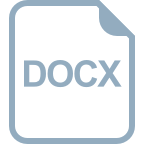
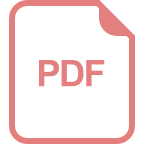















