用c++写一个wss的连接
时间: 2023-03-31 21:00:24 浏览: 200
我可以回答这个问题。要使用 C 语言编写 WebSocket Secure (WSS) 连接,需要使用 OpenSSL 库。以下是一个简单的示例代码:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <netdb.h>
#include <arpa/inet.h>
#include <sys/socket.h>
#include <openssl/ssl.h>
#include <openssl/err.h>
#define SERVER "example.com"
#define PORT 443
int main() {
SSL_CTX *ctx;
SSL *ssl;
int sockfd;
struct sockaddr_in server_addr;
struct hostent *server;
// 初始化 OpenSSL 库
SSL_library_init();
OpenSSL_add_all_algorithms();
SSL_load_error_strings();
// 创建 SSL 上下文
ctx = SSL_CTX_new(TLSv1_2_client_method());
if (ctx == NULL) {
printf("Error creating SSL context\n");
return 1;
}
// 创建 TCP 套接字
sockfd = socket(AF_INET, SOCK_STREAM, );
if (sockfd < ) {
printf("Error creating socket\n");
return 1;
}
// 获取服务器 IP 地址
server = gethostbyname(SERVER);
if (server == NULL) {
printf("Error resolving hostname\n");
return 1;
}
// 设置服务器地址
memset(&server_addr, , sizeof(server_addr));
server_addr.sin_family = AF_INET;
server_addr.sin_port = htons(PORT);
memcpy(&server_addr.sin_addr.s_addr, server->h_addr, server->h_length);
// 连接服务器
if (connect(sockfd, (struct sockaddr *)&server_addr, sizeof(server_addr)) < ) {
printf("Error connecting to server\n");
return 1;
}
// 创建 SSL 对象
ssl = SSL_new(ctx);
if (ssl == NULL) {
printf("Error creating SSL object\n");
return 1;
}
// 将 SSL 对象与套接字关联
SSL_set_fd(ssl, sockfd);
// 建立 SSL 连接
if (SSL_connect(ssl) != 1) {
printf("Error establishing SSL connection\n");
return 1;
}
// 发送数据
char *msg = "Hello, server!";
SSL_write(ssl, msg, strlen(msg));
// 接收数据
char buf[1024];
int len = SSL_read(ssl, buf, sizeof(buf) - 1);
buf[len] = '\';
printf("Received: %s\n", buf);
// 关闭 SSL 连接和套接字
SSL_shutdown(ssl);
SSL_free(ssl);
close(sockfd);
// 清理 OpenSSL 库
ERR_free_strings();
EVP_cleanup();
SSL_CTX_free(ctx);
return ;
}
阅读全文
相关推荐
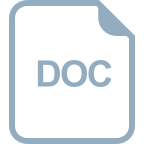
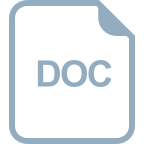
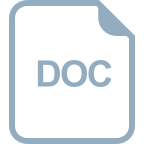
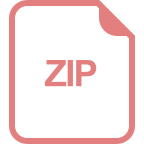
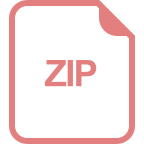
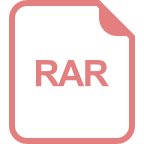
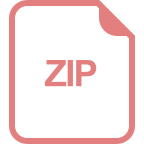
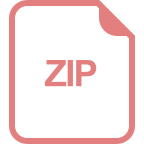
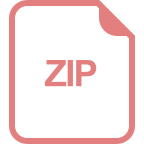









