编写求x的n次方的递归函数,在主函数调用并输出。(x为double型,n为整型,函数类型为double型)
时间: 2024-02-05 10:12:29 浏览: 75
好的,我明白了。以下是相应的代码实现:
```c++
#include <iostream>
using namespace std;
double pow(double x, int n) {
if (n == 0) {
return 1;
}
double t = pow(x, n / 2);
if (n % 2) {
return x * t * t;
} else {
return t * t;
}
}
int main() {
double x;
int n;
cout << "请输入底数x和指数n: ";
cin >> x >> n;
double res = pow(x, n);
cout << x << "的" << n << "次方为:" << res << endl;
return 0;
}
```
以上的代码中,我们使用了递归的方式实现求x的n次方。主函数中输入了底数x和指数n,然后调用了求幂的函数,并输出结果。在函数内部,如果n为0,则返回1;如果n为奇数,则返回x * (t * t);如果n为偶数,则返回t * t。其中,t代表递归计算中的中间值。
相关问题
编写求x的n次方的递归函数,在主函数调用并输出。C语言
好的,我可以帮你编写一个求x的n次方的递归函数,在主函数调用并输出。以下是代码:
```
#include <stdio.h>
double power(double x, int n) {
if (n == 0) {
return 1;
} else {
return x * power(x, n - 1);
}
}
int main() {
double x;
int n;
printf("请输入一个实数x:");
scanf("%lf", &x);
printf("请输入一个整数n:");
scanf("%d", &n);
printf("%lf的%d次方为%lf", x, n, power(x, n));
return 0;
}
```
你可以把这段代码复制到你的编辑器中,然后运行它,输入x和n,就可以求出x的n次方了。如果你有其他问题,欢迎随时问我。
用c语言编写求n!的递归函数,在主函数调用并输出,注意n为整型函数类型为double型
好的,下面是代码:
```c
#include <stdio.h>
double factorial(int n);
int main() {
int n;
printf("请输入n的值:");
scanf("%d", &n);
printf("%d! = %.0f\n", n, factorial(n));
return 0;
}
double factorial(int n) {
if (n == 0) {
return 1.0;
} else {
return n * factorial(n - 1);
}
}
```
首先在程序开头声明了一个`factorial`函数,这样在主函数中调用时就不会出现函数未定义的错误。然后定义了`factorial`函数,与上一个答案中的代码相同。在主函数中读入`n`的值,并调用`factorial`函数计算`n!`的值并输出。
阅读全文
相关推荐
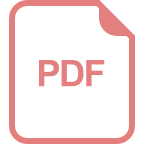
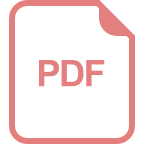












