pyopengl选中物体
时间: 2023-10-16 07:10:30 浏览: 35
要实现选中物体,通常需要进行以下步骤:
1. 确定鼠标点击的位置。
2. 将鼠标点击的位置转换为OpenGL坐标系中的坐标。
3. 遍历场景中的所有物体,并检查哪些物体包含鼠标点击的坐标。
4. 如果找到了包含该坐标的物体,则将其标记为选中状态。
以下是一个基本的选中物体的示例代码:
```
import glfw
from OpenGL.GL import *
from OpenGL.GLU import *
# 定义场景中的物体
objects = [
{'name': 'cube1', 'vertices': [(0, 0, 0), (1, 0, 0), (1, 1, 0), (0, 1, 0), (0, 0, 1), (1, 0, 1), (1, 1, 1), (0, 1, 1)], 'color': (1, 0, 0)},
{'name': 'cube2', 'vertices': [(2, 0, 0), (3, 0, 0), (3, 1, 0), (2, 1, 0), (2, 0, 1), (3, 0, 1), (3, 1, 1), (2, 1, 1)], 'color': (0, 1, 0)},
{'name': 'cube3', 'vertices': [(0, 2, 0), (1, 2, 0), (1, 3, 0), (0, 3, 0), (0, 2, 1), (1, 2, 1), (1, 3, 1), (0, 3, 1)], 'color': (0, 0, 1)}
]
# 定义选中物体的颜色
selected_color = (1, 1, 1)
# 定义选中的物体
selected_object = None
# 选中物体的函数
def select_object(x, y):
global selected_object
viewport = glGetIntegerv(GL_VIEWPORT)
modelview = glGetDoublev(GL_MODELVIEW_MATRIX)
projection = glGetDoublev(GL_PROJECTION_MATRIX)
winx, winy, winz = gluUnProject(x, viewport[3]-y, 0, modelview, projection, viewport)
for obj in objects:
vertices = obj['vertices']
xmin, ymin, zmin = vertices[0]
xmax, ymax, zmax = vertices[0]
for vertex in vertices:
x, y, z = vertex
if x < xmin:
xmin = x
elif x > xmax:
xmax = x
if y < ymin:
ymin = y
elif y > ymax:
ymax = y
if z < zmin:
zmin = z
elif z > zmax:
zmax = z
if winx >= xmin and winx <= xmax and winy >= ymin and winy <= ymax and winz >= zmin and winz <= zmax:
selected_object = obj
obj['color'] = selected_color
else:
obj['color'] = tuple([c*0.5 for c in obj['color']])
# 绘制场景的函数
def draw_scene():
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)
glMatrixMode(GL_MODELVIEW)
glLoadIdentity()
gluLookAt(0, 0, 5, 0, 0, 0, 0, 1, 0)
for obj in objects:
glColor3f(*obj['color'])
glBegin(GL_QUADS)
for vertex in obj['vertices']:
glVertex3f(*vertex)
glEnd()
# 鼠标点击事件处理函数
def mouse_button_callback(window, button, action, mods):
if button == glfw.MOUSE_BUTTON_LEFT and action == glfw.PRESS:
x, y = glfw.get_cursor_pos(window)
select_object(x, y)
# 主函数
def main():
if not glfw.init():
return
window = glfw.create_window(640, 480, 'Select Object', None, None)
if not window:
glfw.terminate()
return
glfw.make_context_current(window)
glfw.set_mouse_button_callback(window, mouse_button_callback)
glEnable(GL_DEPTH_TEST)
while not glfw.window_should_close(window):
draw_scene()
glfw.swap_buffers(window)
glfw.poll_events()
glfw.terminate()
if __name__ == '__main__':
main()
```
该示例代码定义了三个立方体物体,并将它们分别绘制为红色、绿色和蓝色。当用户点击鼠标左键时,程序将检查鼠标点击的位置,如果该位置包含在某个立方体中,则将该立方体的颜色改为白色,表示该立方体被选中了。如果用户点击另一个立方体,则前一个选中的立方体的颜色将被还原为原来的颜色,新选中的立方体的颜色将被改为白色。
相关推荐
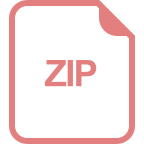














