把最大轮廓重新画在和原图大小一样的白色画布上python 代码
时间: 2024-10-12 22:05:39 浏览: 12
要在Python中使用OpenCV(cv2库)把检测到的最大轮廓重新绘制在与原始图像同样大小的白色画布上,你可以使用以下步骤:
首先,确保已经安装了`opencv-python`库。如果没有安装,可以使用pip安装:
```bash
pip install opencv-python
```
然后,这是一个简单的示例代码,展示如何处理这个问题:
```python
import cv2
import numpy as np
# 加载图像
image = cv2.imread('input_image.jpg')
# 转换为灰度图像以便于边缘检测
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 应用阈值处理获取二进制图像
_, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 寻找轮廓
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 找到最大的轮廓
if len(contours) > 0:
max_contour = max(contours, key=cv2.contourArea)
else:
print("No contours found.")
return
# 计算轮廓的外接矩形
x, y, w, h = cv2.boundingRect(max_contour)
# 创建一个新的白色背景的画布,尺寸与原图相同
canvas_width, canvas_height = image.shape[:2]
white_canvas = np.zeros((canvas_height, canvas_width), dtype=np.uint8).astype(np.uint8)*255
# 将最大轮廓重新绘制在白色画布上
cv2.drawContours(white_canvas, [max_contour], -1, (255, 255, 255), -1)
# 如果需要,可以调整绘制位置
# white_canvas[y:y+h, x:x+w] = image[y:y+h, x:x+w]
# 显示结果
cv2.imshow('Original', image)
cv2.imshow('Canvas with Max Contour', white_canvas)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
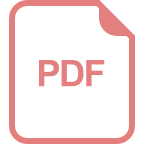
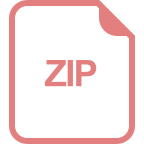

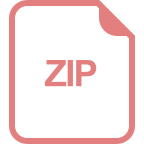
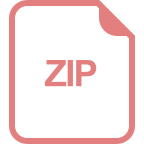
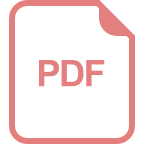
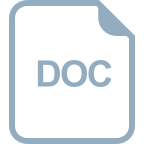
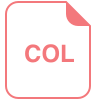
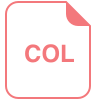
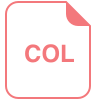


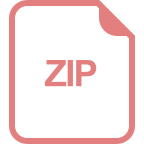
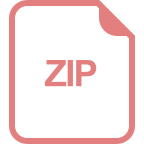
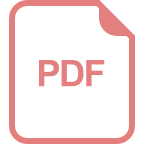