用c语言写一个图像灰度化算法
时间: 2024-05-15 14:12:26 浏览: 22
下面是一个简单的C语言代码示例,实现将彩色图像转换为灰度图像的算法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#define STB_IMAGE_IMPLEMENTATION
#include "stb_image.h"
#define STB_IMAGE_WRITE_IMPLEMENTATION
#include "stb_image_write.h"
int main(int argc, char** argv) {
if (argc < 3) {
printf("Usage: %s input_image output_image\n", argv[0]);
return 1;
}
const char* input_filename = argv[1];
const char* output_filename = argv[2];
int width, height, channels;
unsigned char *image = stbi_load(input_filename, &width, &height, &channels, 0);
if (!image) {
printf("Error loading image %s\n", input_filename);
return 1;
}
unsigned char *gray_image = (unsigned char*)malloc(width * height);
if (!gray_image) {
printf("Error allocating memory\n");
return 1;
}
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
int index = (i * width + j) * channels;
int r = image[index];
int g = image[index + 1];
int b = image[index + 2];
// 灰度化处理公式
gray_image[i * width + j] = 0.299 * r + 0.587 * g + 0.114 * b;
}
}
stbi_write_png(output_filename, width, height, 1, gray_image, 0);
printf("Gray image saved to %s\n", output_filename);
stbi_image_free(image);
free(gray_image);
return 0;
}
```
该程序使用了stb_image和stb_image_write库来加载和保存图像,您需要将这些库的头文件和源文件添加到您的项目中。该程序将彩色图像转换为灰度图像的方式是使用常见的加权平均法,即将每个像素的红、绿、蓝三个通道的值按照一定比例加权求和得到灰度值。
相关推荐
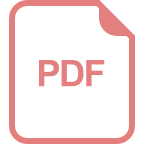
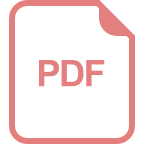














