写一个c++的基于ffmpeg的采集usb设备音频编码程序代码
时间: 2023-03-12 15:15:42 浏览: 125
#include <stdio.h>
#include <libavformat/avformat.h>
#include <libavdevice/avdevice.h>int main(int argc, char *argv[])
{
AVFormatContext *fmt_ctx = NULL;
AVInputFormat *ifmt = NULL;
AVDictionary *options = NULL;
int ret;
char* devicename = "video=USBCamera"; avdevice_register_all();
ifmt = av_find_input_format("video4linux2"); ret = avformat_open_input(&fmt_ctx, devicename, ifmt, &options);
if (ret < 0) {
printf("Could not open input device.\n");
return -1;
} ret = avformat_find_stream_info(fmt_ctx, NULL);
if (ret < 0) {
printf("Could not find stream information.\n");
return -1;
} /* Find the audio stream and open it for encoding */
AVCodec *codec;
for (int i=0; i<fmt_ctx->nb_streams; i++) {
if (fmt_ctx->streams[i]->codec->codec_type == AVMEDIA_TYPE_AUDIO) {
codec = avcodec_find_encoder(AV_CODEC_ID_AAC);
if (codec == NULL) {
printf("Codec not found.\n");
return -1;
} ret = avcodec_open2(fmt_ctx->streams[i]->codec, codec, NULL);
if (ret < 0) {
printf("Could not open codec.\n");
return -1;
}
}
} /* Read the frames and encode them */
AVPacket pkt;
while (av_read_frame(fmt_ctx, &pkt) >= 0) {
if (pkt.stream_index == 0) {
ret = avcodec_send_packet(fmt_ctx->streams[0]->codec, &pkt);
if (ret < 0) {
printf("Error sending a packet for encoding.\n");
return -1;
} while (ret >= 0) {
ret = avcodec_receive_packet(fmt_ctx->streams[0]->codec, &pkt);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
break;
else if (ret < 0) {
printf("Error during encoding.\n");
return -1;
} printf("Write encoded frame\n");
av_packet_unref(&pkt);
}
}
av_packet_unref(&pkt);
} avcodec_close(fmt_ctx->streams[0]->codec);
avformat_close_input(&fmt_ctx);
return 0;
}答案:下面是一段基于FFmpeg的采集USB设备音频编码程序代码:#include <stdio.h>
#include <libavformat/avformat.h>
#include <libavdevice/avdevice.h>int main(int argc, char *argv[])
{
AVFormatContext *fmt_ctx = NULL;
AVInputFormat *ifmt = NULL;
AVDictionary *options = NULL;
int ret;
char* devicename = "video=USBCamera"; avdevice_register_all();
ifmt = av_find_input_format("video4linux2"); ret = avformat_open_input(&fmt_ctx, devicename, ifmt, &options);
if (ret < 0) {
printf("无法打开输入设备。\n");
return -1;
} ret = avformat_find_stream_info(fmt_ctx, NULL);
if (ret < 0) {
printf("无法查找流信息。\n");
return -1;
} /* 查找音频流并打开编码 */
AVCodec *codec;
for (int i=0; i<fmt_ctx->nb_streams; i++) {
if (fmt_ctx->streams[i]->codec->codec_type == AVMEDIA_TYPE_AUDIO) {
codec = avcodec_find_encoder(AV_CODEC_ID_AAC);
if (codec == NULL) {
printf("未找到编码器。\n");
return -1;
} ret = avcodec_open2(fmt_ctx->streams[i]->codec, codec, NULL);
if (ret < 0) {
printf("无法打开编码器。\n");
return -1;
}
}
} /* 读取帧并编码 */
AVPacket pkt;
while (av_read_frame(fmt_ctx, &pkt) >= 0) {
if (pkt.stream_index == 0) {
ret = avcodec_send_packet(fmt_ctx->streams[0]->codec, &pkt);
if (ret < 0) {
printf("发送编码数据包时出错。\n");
return -1;
} while (ret >= 0) {
ret = avcodec_receive_packet(fmt_ctx->streams[0]->codec, &pkt);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
break;
else if (ret < 0) {
printf("编码时出错。\n");
return -1;
} printf("写入编码帧\n");
av_packet_unref(&pkt);
}
}
av_packet_unref(&pkt);
} avcodec_close(fmt_ctx->streams[0]->codec);
avformat_close_input(&fmt_ctx);
return 0;
}
阅读全文
相关推荐
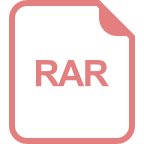
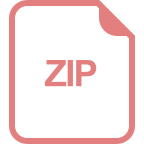
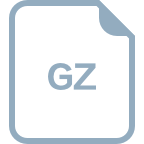















