c++平方数可计算复数计算器
时间: 2023-08-03 21:35:47 浏览: 51
您好,我可以帮助您解决这个问题。您需要使用C++的复数类,例如std::complex。下面是一个简单的示例代码,可以计算两个复数的平方和。
```c++
#include <iostream>
#include <complex>
using namespace std;
int main() {
// 定义两个复数
complex<double> z1(3.0, 4.0); // 3+4i
complex<double> z2(1.0, 2.0); // 1+2i
// 计算两个复数的平方和
complex<double> z3 = pow(z1, 2) + pow(z2, 2);
// 输出结果
cout << "The square sum of " << z1 << " and " << z2 << " is " << z3 << endl;
return 0;
}
```
在这个示例中,我们使用了C++的std::complex库来定义两个复数,然后使用pow函数计算它们的平方,最后将结果相加得到一个新的复数。您可以根据自己的需要修改代码,以实现您的复数计算器。
相关问题
c++复数计算器设计报告
本报告将介绍使用C++语言设计复数计算器的过程和实现细节。本报告将分为以下几个部分:
1. 需求分析
2. 设计思路
3. 实现方法
4. 测试和性能优化
## 1. 需求分析
复数计算器需要支持以下基本操作:
- 复数加法
- 复数减法
- 复数乘法
- 复数除法
- 复数的模
- 复数的共轭
为了方便使用,我们还需要支持以下附加功能:
- 支持输入输出
- 支持任意精度运算
## 2. 设计思路
为了实现复数的运算,我们需要设计一个复数类,同时重载运算符以方便使用。在设计复数类时,我们需要考虑的因素包括:
- 复数的实部和虚部
- 复数的加减乘除运算
- 复数的模和共轭
- 复数的输入输出
- 复数的精度
为了方便使用,我们还可以设计一个控制台界面来与用户交互,同时可以通过命令行参数来指定精度等设置。
## 3. 实现方法
### 复数类
我们首先定义一个复数类,其中包括实部和虚部两个成员变量,以及一些成员函数用于实现复数的基本操作。
```cpp
class Complex {
public:
Complex(double real = 0, double imag = 0);
double getReal() const;
double getImag() const;
double getMod() const;
Complex getConjugate() const;
Complex operator+(const Complex &rhs) const;
Complex operator-(const Complex &rhs) const;
Complex operator*(const Complex &rhs) const;
Complex operator/(const Complex &rhs) const;
friend std::ostream &operator<<(std::ostream &os, const Complex &c);
friend std::istream &operator>>(std::istream &is, Complex &c);
private:
double real_;
double imag_;
};
```
其中,构造函数用于初始化实部和虚部,getReal()、getImag()、getMod()、getConjugate()分别用于获取实部、虚部、模和共轭。
重载的运算符包括加减乘除四个基本运算符,以及输入输出运算符。
### 控制台界面
我们可以通过命令行参数来指定精度等设置,同时设计一个控制台界面来与用户交互。
```cpp
int main(int argc, char *argv[]) {
int precision = 2;
if (argc > 1) {
precision = std::atoi(argv[1]);
}
std::cout << "Welcome to Complex Calculator!" << std::endl;
std::cout << "Set precision to " << precision << std::endl;
std::string line;
while (std::getline(std::cin, line)) {
if (line.empty()) {
continue;
}
std::istringstream iss(line);
Complex c1, c2;
std::string op;
iss >> c1 >> op >> c2;
if (!iss) {
std::cout << "Invalid input!" << std::endl;
continue;
}
Complex result;
if (op == "+") {
result = c1 + c2;
} else if (op == "-") {
result = c1 - c2;
} else if (op == "*") {
result = c1 * c2;
} else if (op == "/") {
result = c1 / c2;
} else {
std::cout << "Invalid operator: " << op << std::endl;
continue;
}
std::cout << std::fixed << std::setprecision(precision) << result << std::endl;
}
return 0;
}
```
## 4. 测试和性能优化
为了测试复数计算器的正确性和性能,我们可以编写一些测试用例,并使用Valgrind等工具来检查内存泄漏等问题。在实际使用中,我们还可以通过多线程等技术来提高计算器的性能。
## 总结
本报告介绍了使用C++语言设计复数计算器的过程和实现细节,包括复数类的设计、控制台界面的实现、测试和性能优化等方面。复数计算器对于科学计算、工程计算等领域具有重要的应用价值,本报告提供了一种简单易用的实现方法。
c++重载函数复数计算器
下面是一个简单的C++复数计算器,其中重载了加法、减法、乘法和除法运算符,可以进行复数的加减乘除运算。
```c++
#include <iostream>
using namespace std;
class Complex
{
private:
double real;
double imag;
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(const Complex& other) const
{
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const
{
return Complex(real - other.real, imag - other.imag);
}
Complex operator*(const Complex& other) const
{
return Complex(real * other.real - imag * other.imag, real * other.imag + imag * other.real);
}
Complex operator/(const Complex& other) const
{
double denominator = other.real * other.real + other.imag * other.imag;
return Complex((real * other.real + imag * other.imag) / denominator, (imag * other.real - real * other.imag) / denominator);
}
friend ostream& operator<<(ostream& os, const Complex& c)
{
os << c.real << "+" << c.imag << "i";
return os;
}
};
int main()
{
Complex a(2, 3);
Complex b(4, 5);
cout << "a = " << a << endl;
cout << "b = " << b << endl;
cout << "a + b = " << a + b << endl;
cout << "a - b = " << a - b << endl;
cout << "a * b = " << a * b << endl;
cout << "a / b = " << a / b << endl;
return 0;
}
```
在上面的代码中,我们定义了一个名为Complex的类,它有两个私有成员变量real和imag,表示实部和虚部。我们定义了一个构造函数,用于初始化实部和虚部。
然后我们重载了加法、减法、乘法和除法运算符,使得我们可以像操作普通数字一样操作复数。在重载运算符时,我们返回一个新的Complex对象,其中包含了运算结果。
最后,我们定义了一个友元函数operator<<,用于将Complex对象输出到流中,以便我们可以在控制台中查看运算结果。
相关推荐
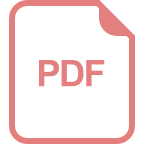
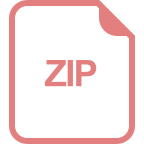
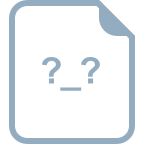












