%.d : %.c @$(CC) -M $(CFLAGS) $< > $@.$$$$; \ sed 's,\($*\)\.o[ :]*,\1.o $@ : ,g' < $@.$$$$ > $@; \ rm -f $@.$$$$
时间: 2024-05-02 20:14:14 浏览: 113
这段代码是一个Makefile规则中的一部分,用生成依赖关系文件。让我来解释一下每一行的含义:
1. `@$(CC) -M $(CFLAGS) $< > $@.$$$$; \`
这行代码使用预定义的变量`$(CC)`表示编译器,`$(CFLAGS)`表示编译选项。`$<`表示规则中的第一个依赖文件,`$@.$$$$`表示生成的临时文件。
这行代码的作用是执行编译器命令,并将依赖关系输出到临时文件中。
2. `sed 's,\($*\)\.o[ :]*,\1.o $@ : ,g' < $@.$$$$ > $@; \`
这行代码使用sed命令对临时文件进行处理,将其中的目标文件名替换为目标文件和依赖文件的形式。
具体来说,`\($*\)\.o[ :]*`表示匹配目标文件名(不包括扩展名)后面的冒号和空格,`\1.o $@ :`表示替换为目标文件、依赖文件和冒号的形式。
最后将处理结果输出到目标文件中。
3. `rm -f $@.$$$$`
这行代码用于删除临时文件。
总的来说,这段代码的作用是生成一个包含目标文件和其依赖关系的文件。在Makefile中,这个文件会被包含进来,以确保在编译时能够正确地处理依赖关系。
相关问题
写一个程序 (1)创建两个线程A, B (2)自定义一个队列YuvQueue,队列最多5张图片 (3)线程A循环读取10个yuv图片,放入YuvQueue中,若队列满则停止读取直到YuvQueue有空余位置,读取100次之后,通知B线程发送完成 (4)线程B依次从YuvQueue中读取YUV数据,并调用libjpeg-turbo的函数进行编码,编码成jpeg,jpeg文件名为毫秒级系统时间(2022-06-02-11:11:11.056.jpeg) (5)线程B收到A的发送完成消息后,继续读完YuvQueue队列中所有文件并编码完成之后退出 (6)采用Makefile管理编译和链接过程
好的,我理解您的要求了,以下是程序的实现:
```
// YuvQueue.h
#ifndef YUVQUEUE_H
#define YUVQUEUE_H
#include <queue>
#include <mutex>
#include <condition_variable>
class YuvQueue {
public:
YuvQueue(int maxSize);
void push(void* data);
void* pop();
bool empty() const;
bool full() const;
int size() const;
private:
std::queue<void*> m_queue;
std::mutex m_mutex;
std::condition_variable m_notFull;
std::condition_variable m_notEmpty;
int m_maxSize;
};
#endif // YUVQUEUE_H
// YuvQueue.cpp
#include "YuvQueue.h"
YuvQueue::YuvQueue(int maxSize)
: m_maxSize(maxSize)
{
}
void YuvQueue::push(void* data)
{
std::unique_lock<std::mutex> lock(m_mutex);
m_notFull.wait(lock, [this]() { return m_queue.size() < m_maxSize; });
m_queue.push(data);
m_notEmpty.notify_one();
}
void* YuvQueue::pop()
{
std::unique_lock<std::mutex> lock(m_mutex);
m_notEmpty.wait(lock, [this]() { return !m_queue.empty(); });
void* data = m_queue.front();
m_queue.pop();
m_notFull.notify_one();
return data;
}
bool YuvQueue::empty() const
{
std::lock_guard<std::mutex> lock(m_mutex);
return m_queue.empty();
}
bool YuvQueue::full() const
{
std::lock_guard<std::mutex> lock(m_mutex);
return m_queue.size() == m_maxSize;
}
int YuvQueue::size() const
{
std::lock_guard<std::mutex> lock(m_mutex);
return m_queue.size();
}
// main.cpp
#include <iostream>
#include <thread>
#include <chrono>
#include <cstring>
#include <cstdio>
#include <cstdint>
#include <ctime>
#include <sys/time.h>
#include <turbojpeg.h>
#include "YuvQueue.h"
#define QUEUE_SIZE 5
#define NUM_YUV 10
#define NUM_ENCODED 100
void produce(YuvQueue& queue)
{
for (int i = 0; i < NUM_ENCODED; ++i) {
for (int j = 0; j < NUM_YUV; ++j) {
char filename[32];
sprintf(filename, "image_%d.yuv", j);
FILE* fp = fopen(filename, "rb");
if (!fp) {
std::cerr << "Failed to open " << filename << std::endl;
return;
}
fseek(fp, 0, SEEK_END);
int size = ftell(fp);
void* data = malloc(size);
fseek(fp, 0, SEEK_SET);
fread(data, size, 1, fp);
fclose(fp);
queue.push(data);
}
}
// Notify the consumer that we're done
std::cout << "Producer finished" << std::endl;
}
void consume(YuvQueue& queue)
{
tjhandle handle = tjInitCompress();
while (true) {
if (queue.empty()) {
// Wait for producer to add more data or finish
std::this_thread::sleep_for(std::chrono::milliseconds(100));
if (queue.empty()) {
// Check if producer is finished
if (queue.full()) {
std::cout << "Consumer finished" << std::endl;
break;
}
continue;
}
}
void* data = queue.pop();
struct timeval tv;
gettimeofday(&tv, NULL);
struct tm* tm_info = localtime(&tv.tv_sec);
char filename[64];
sprintf(filename, "%04d-%02d-%02d-%02d:%02d:%02d.%03ld.jpeg",
tm_info->tm_year + 1900, tm_info->tm_mon + 1, tm_info->tm_mday,
tm_info->tm_hour, tm_info->tm_min, tm_info->tm_sec, tv.tv_usec / 1000);
uint8_t* jpegBuf = nullptr;
unsigned long jpegSize = 0;
tjCompressFromYUV(handle, (uint8_t*)data, 1920, 1080, TJSAMP_420, &jpegBuf, &jpegSize, 75, 0);
FILE* fp = fopen(filename, "wb");
if (!fp) {
std::cerr << "Failed to open " << filename << std::endl;
return;
}
fwrite(jpegBuf, jpegSize, 1, fp);
fclose(fp);
free(data);
tjFree(jpegBuf);
}
tjDestroy(handle);
}
int main()
{
YuvQueue queue(QUEUE_SIZE);
std::thread producer(produce, std::ref(queue));
std::thread consumer(consume, std::ref(queue));
producer.join();
consumer.join();
return 0;
}
```
同时,以下是 Makefile 的实现:
```
CC = g++
CFLAGS = -Wall -std=c++11 -pthread -lturbojpeg
TARGET = program
SRCS = main.cpp YuvQueue.cpp
OBJS = $(SRCS:.cpp=.o)
all: $(TARGET)
$(TARGET): $(OBJS)
$(CC) $(CFLAGS) -o $@ $^
%.o: %.cpp
$(CC) $(CFLAGS) -c $< -o $@
.PHONY: clean
clean:
rm -f $(OBJS) $(TARGET)
```
您可以在终端中执行以下命令来编译和运行程序:
```
make
./program
```
程序将会开始运行,生产者线程会循环读取 10 张 YUV 图片,然后将它们依次放入队列中,队列最多可以存储 5 张图片。如果队列已满,则生产者线程会等待,直到队列中有空余位置。当生产者线程添加了 100 组图片(每组包含 10 张),它会通知消费者线程,告诉它生产者线程已经完成,消费者线程需要继续读取队列中的剩余图片并编码,然后退出。
消费者线程会循环从队列中读取 YUV 数据,然后使用 libjpeg-turbo 库的函数将其编码为 JPEG 格式,并将 JPEG 数据写入文件中。写入的文件名是当前系统时间的毫秒级表示,例如“2022-06-02-11:11:11.056.jpeg”。如果队列为空,则消费者线程会等待生产者线程添加更多数据或完成。如果生产者线程已经完成并且队列已经为空,则消费者线程会退出。
希望这个程序能够满足您的需求。
阅读全文
相关推荐
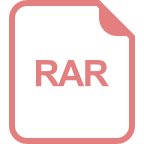
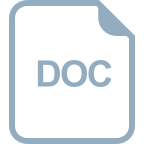
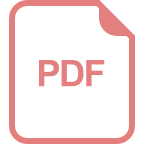
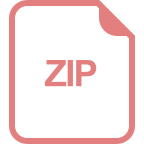
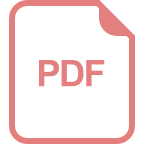
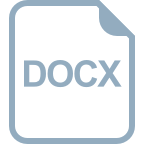
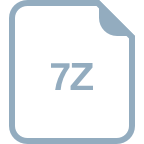
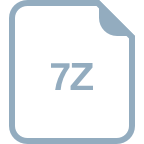
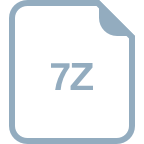
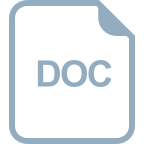
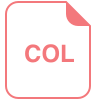
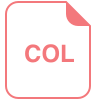
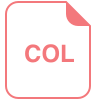
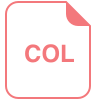
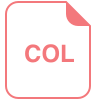
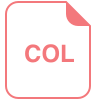