VUE微信小程序实现购物车代码
时间: 2024-12-14 18:16:58 浏览: 5
Vue.js可以用于构建微信小程序,但微信小程序有自己的框架和API,并不直接支持Vue。如果你想在微信小程序中实现购物车功能,你需要结合微信小程序提供的`wx.request`进行数据交互,利用组件化思想管理商品列表和购物车状态。
以下是一个简化的示例,展示了如何创建一个基础的购物车功能:
1. **商品列表组件 (`goods-list.vue`)**:
```html
<template>
<view>
<view class="item" v-for="(good, index) in goods" :key="index">
<text>{{ good.name }}</text>
<button @click=" addToCart(good)">加入购物车</button>
</view>
</view>
</template>
<script>
export default {
data() {
return {
goods: [], // 商品数组
};
},
methods: {
addToCart(good) {
this.$emit('cart-add', good);
},
},
};
</script>
```
2. **购物车组件 (`shopping-cart.vue`)**:
```html
<template>
<view>
<view>购物车:</view>
<view v-for="(item, index) in cartItems" :key="index">{{ item.name }} - {{ item.price }}</view>
</view>
</template>
<script>
import { mapState } from 'vuex';
export default {
computed: {
...mapState(['cartItems']), // 获取store中的购物车状态
},
methods: {
handleCartAdd(item) {
this.cartItems.push(item);
// 这里需要处理实际添加到微信小程序购物车的数据存储或请求
},
},
};
</script>
```
3. **Vuex store (store.js)**:
```js
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
const state = {
cartItems: [],
};
const mutations = {
addItemToCart(state, item) {
state.cartItems.push(item);
},
};
export default new Vuex.Store({
state,
mutations,
});
```
4. **在主页面 (`app.wxss` 和 `pages/index/index.wxml` 中引用组件并连接它们)**:
将这两个组件嵌套在小程序的页面结构中,并通过事件总线(`$emit` 和 `$on`)在两者之间传递数据。
这只是一个基本示例,实际应用中还需要考虑错误处理、商品库存检查、以及与微信小程序官方提供的支付接口集成等复杂情况。记住,微信小程序有自己的限制,如不允许使用DOM操作等,所以开发时应遵循其规则。
阅读全文
相关推荐
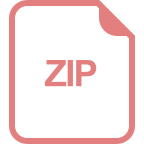
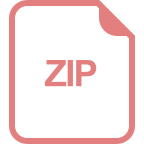
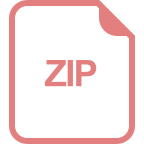
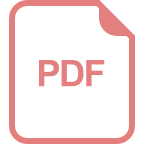
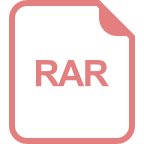
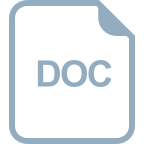
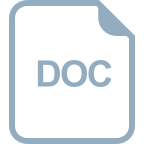


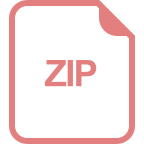
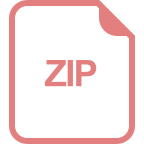
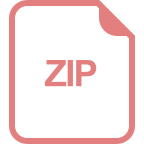
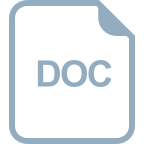
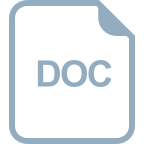
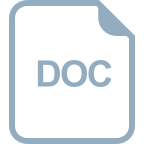
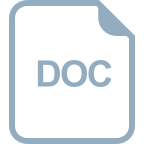
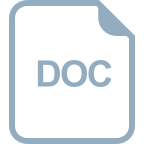
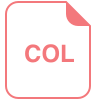
