对有序数据表(5,7,9,12,15,18,20,22,25,30,100),编写程序按折半查找方法查找12和28。C语言代码实现
时间: 2023-09-20 11:11:40 浏览: 95
下面是用 C 语言实现折半查找算法的代码:
```c
#include <stdio.h>
int binary_search(int arr[], int left, int right, int target) {
if (left > right) {
return -1; // 没有找到
}
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid; // 找到了,返回下标
} else if (arr[mid] > target) {
return binary_search(arr, left, mid - 1, target); // 在左侧查找
} else {
return binary_search(arr, mid + 1, right, target); // 在右侧查找
}
}
int main() {
int arr[] = {5, 7, 9, 12, 15, 18, 20, 22, 25, 30, 100};
int n = sizeof(arr) / sizeof(arr[0]);
int target1 = 12;
int target2 = 28;
int idx1 = binary_search(arr, 0, n - 1, target1);
if (idx1 == -1) {
printf("%d not found in the array.\n", target1);
} else {
printf("%d is found at index %d.\n", target1, idx1);
}
int idx2 = binary_search(arr, 0, n - 1, target2);
if (idx2 == -1) {
printf("%d not found in the array.\n", target2);
} else {
printf("%d is found at index %d.\n", target2, idx2);
}
return 0;
}
```
输出结果:
```
12 is found at index 3.
28 not found in the array.
```
阅读全文
相关推荐
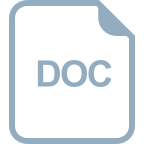
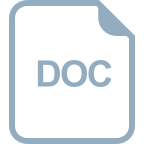
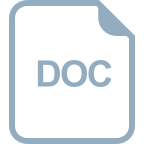
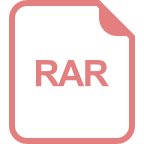
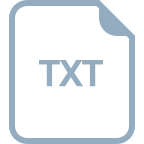
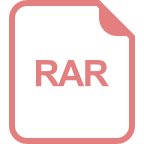
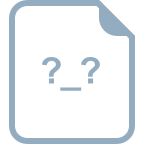
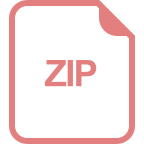
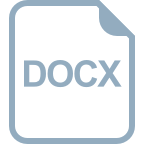
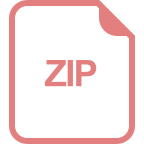
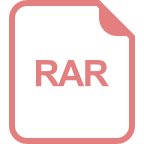
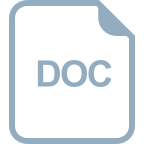
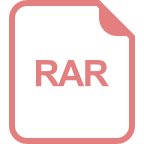
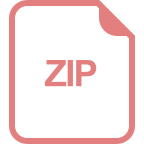
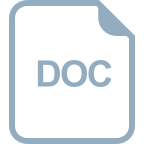
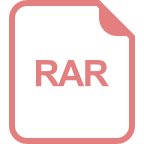
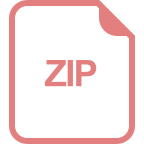
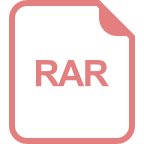
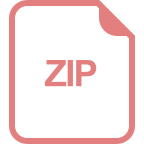