contours, hierarchy = cv2.findContours(binary, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
时间: 2024-05-09 12:04:06 浏览: 96
这行代码使用 OpenCV 的 `findContours` 函数从二值化图像中提取轮廓。它接受三个参数:
- `binary`:二值化的输入图像。
- `cv2.RETR_EXTERNAL`:表示只提取最外层轮廓。
- `cv2.CHAIN_APPROX_SIMPLE`:表示压缩水平、垂直和对角线方向上相邻的轮廓点,只保留端点。
函数的返回值是一个包含两个元素的元组:轮廓列表和轮廓的层次结构。其中,轮廓列表是一个包含每个轮廓点坐标的 numpy 数组的列表,而轮廓的层次结构是一个 numpy 数组,描述了每个轮廓之间的关系。
相关问题
import cv2import numpy as np# 读取图像image = cv2.imread('工件图像.jpg')# 转换为灰度图像gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)# 使用二值化处理获取缺陷图像ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)# 使用形态学操作进行缺陷的去噪和填充kernel = np.ones((3,3), np.uint8)closing = cv2.morphologyEx(thresh, cv2.MORPH_CLOSE, kernel)opening = cv2.morphologyEx(closing, cv2.MORPH_OPEN, kernel)# 获取缺陷轮廓contours, hierarchy = cv2.findContours(opening, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)# 遍历每一个缺陷,提取几何特征并绘制位置for i in range(len(contours)): # 获取面积和周长 area = cv2.contourArea(contours[i]) perimeter = cv2.arcLength(contours[i], True) # 获取质心 moments = cv2.moments(contours[i]) cx = int(moments['m10'] / moments['m00']) cy = int(moments['m01'] / moments['m00']) # 绘制位置和特征 cv2.drawContours(image, [contours[i]], -1, (0, 255, 0), 2) cv2.circle(image,(cx,cy), 5, (0,0,255), -1) cv2.putText(image, "Area: {:.2f}".format(area), (cx+10, cy-10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (255,255,255), 2) cv2.putText(image, "Perimeter: {:.2f}".format(perimeter), (cx+10, cy+10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (255,255,255), 2)# 显示结果cv2.imshow('Defect Detection', image)cv2.waitKey(0)# 保存结果cv2.imwrite('Defect Detection.jpg', image)
这是Python中的两个库,cv2是OpenCV库,用于图像和视频处理,包括读取、写入、显示、处理、分析等。numpy是一个数学库,用于处理数字和数组。一般情况下,当我们读取图像时,使用cv2,而对图片进行处理时,使用numpy。
contours, _ = cv.findContours(binary1, cv.RETR_TREE, cv.CHAIN_APPROX_SIMPLE)
This line of code uses the OpenCV library to find contours in a binary image.
- The first argument, "binary1", is the input image. It should be a binary image with white objects on a black background.
- The second argument, "cv.RETR_TREE", specifies the retrieval mode for contours. This mode retrieves all of the contours and reconstructs a full hierarchy of nested contours.
- The third argument, "cv.CHAIN_APPROX_SIMPLE", specifies the contour approximation method. This method compresses horizontal, vertical, and diagonal segments and leaves only their end points. For example, a rectangle contour would be represented by only 4 points instead of a series of connected lines.
The function returns two values:
- "contours" is a list of all the contours found in the image, each represented as a list of points.
- The second value is not assigned to anything, so it is discarded. It represents the hierarchy of contours, which is useful for understanding how contours are nested within each other.
阅读全文
相关推荐
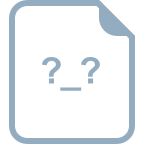














