如何使用C++实现迭代法来计算实数a的平方,要求每次迭代后新旧根之差不超过10^-5?迭代公式为 Xn+1 = (Xn + a / Xn) / 2,请给出相应的C++代码实现步骤和示例。
时间: 2024-11-20 18:56:02 浏览: 44
在C++中,你可以使用迭代法(也称为牛顿法或二分法的一种变种)通过循环来逼近a的平方根。为了保证每次迭代后的误差小于10^-5,我们可以设置一个判断条件来检查当前的近似值与前一次的差距是否满足这个精度。
以下是一个简单的C++代码实现步骤:
1. 首先,声明变量`oldRoot`和`newRoot`存储上一轮和当前的估计值,以及一个变量`epsilon`作为允许的最大误差(这里设为1e-5)。
2. 初始化`oldRoot`为a的一个初始猜测值,通常可以选择a本身或者1,这里我们选择1。
3. 使用while循环,当`newRoot - oldRoot`的绝对值大于`epsilon`时,继续迭代。
4. 计算`newRoot`,即`(oldRoot + a / oldRoot) / 2`。
5. 更新`oldRoot`为`newRoot`。
6. 当满足误差条件时,跳出循环并返回`newRoot`作为结果。
以下是对应的C++代码实现:
```cpp
#include <cmath>
#include <iostream>
double sqrtApproximation(double a, double epsilon = 1e-5)
{
if (a <= 0)
throw std::runtime_error("Cannot calculate square root of non-positive number.");
double oldRoot = 1.0; // Initial guess for the square root
double newRoot;
while (std::abs(newRoot - oldRoot) > epsilon)
{
newRoot = (oldRoot + a / oldRoot) / 2.0;
oldRoot = newRoot;
}
return newRoot;
}
int main()
{
double a = 9; // Example value to find its square root
try
{
double result = sqrtApproximation(a);
std::cout << "The square root of " << a << " is approximately: " << result << std::endl;
}
catch (const std::exception& e)
{
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
阅读全文
相关推荐




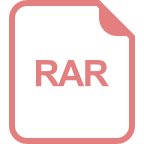













