winpcap 高性能批量发送数据,使用c++编写实例
时间: 2024-12-17 17:38:54 浏览: 19
WinPcap是一个强大的网络包捕获和分析软件库,它允许用户在Windows平台上高效地抓取网络数据包,并在C++等语言中进行处理。若想利用WinPcap进行高性能批量发送数据,首先需要安装WinPcap库,然后通过其API编写C++程序。
下面是一个简单的C++示例,展示如何使用WinPcap发送ARP请求(一种常见的数据包类型):
```cpp
#include <pcap.h>
#include <stdlib.h>
#include <string.h>
// 定义发送的数据结构
struct ARPRequest {
struct ether_addr src_mac; // 源MAC地址
struct ether_addr dst_mac; // 目标MAC地址
u_short eth_type; // 数据链路层协议类型
};
int main(int argc, char **argv) {
// 初始化WinPcap
pcap_t *handle = pcap_open_live("eth0", BUFSIZ, 1, -1, NULL); // "eth0"替换为你的网卡名
if (handle == NULL) {
printf("Failed to open the capture device.\n");
return -1;
}
// 创建ARP请求
ARPRequest arp_request;
memset(&arp_request.src_mac, 0, sizeof(arp_request.src_mac));
// 设置源MAC地址
ether_addr(mac2bin("your_source_mac"), &arp_request.src_mac);
memset(&arp_request.dst_mac, 0, sizeof(arp_request.dst_mac));
// 设置目标MAC地址
ether_addr(mac2bin("your_target_mac"), &arp_request.dst_mac);
arp_request.eth_type = htons(ETH_P_ARP);
// 发送数据包
const struct pcap_pkthdr header = {0};
char packet[ETH_HLEN + ARP_HLEN];
bpf_mkarp((u_char *)&packet[ETH_HLEN], &header, &arp_request);
int packets_sent = pcap_sendpacket(handle, packet, sizeof(packet));
if (packets_sent < 0) {
printf("Error sending packet: %s\n", pcap_geterr(handle));
} else {
printf("Sent %d ARP requests.\n", packets_sent);
}
// 关闭设备
pcap_close(handle);
return 0;
}
阅读全文
相关推荐












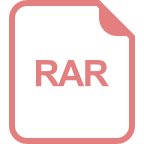



