定义一个基类车,包含轮子,长度,颜色,速度等基本数据成员,可通过构造函数完成初始化;定义基类轮船,包含长度,高度,颜色,速度等基本数据成员,通过构造函数完成初始化操作,定义派生类,水路两用汽车,具备两个基类共有特征,并增添品牌等新数据成员,请定义基类与派生类,并完成基类不低于一个普通成员函数,派生类不低于2个普通成员函数,完成在main函数中的调用。采用普通继承与虚基类继承方式分别完成,并做比较
时间: 2023-06-19 10:05:04 浏览: 69
普通继承方式实现:
```cpp
#include <iostream>
using namespace std;
class Vehicle {
protected:
int wheels;
int length;
string color;
int speed;
public:
Vehicle(int w, int l, string c, int s) : wheels(w), length(l), color(c), speed(s) {}
void showInfo() {
cout << "Wheels: " << wheels << endl;
cout << "Length: " << length << endl;
cout << "Color: " << color << endl;
cout << "Speed: " << speed << endl;
}
};
class Ship {
protected:
int length;
int height;
string color;
int speed;
public:
Ship(int l, int h, string c, int s) : length(l), height(h), color(c), speed(s) {}
void showInfo() {
cout << "Length: " << length << endl;
cout << "Height: " << height << endl;
cout << "Color: " << color << endl;
cout << "Speed: " << speed << endl;
}
};
class AmphibiousVehicle : public Vehicle, public Ship {
private:
string brand;
public:
AmphibiousVehicle(int w, int l, string c, int s, int h, string cc, string b) :
Vehicle(w, l, c, s), Ship(l, h, cc, s), brand(b) {}
void showInfo() {
cout << "Wheels: " << wheels << endl;
cout << "Length: " << length << endl;
cout << "Color: " << color << endl;
cout << "Speed: " << speed << endl;
cout << "Height: " << height << endl;
cout << "Brand: " << brand << endl;
}
void drive() {
cout << "Driving on land." << endl;
}
void sail() {
cout << "Sailing on water." << endl;
}
};
int main() {
AmphibiousVehicle av(4, 6, "Red", 60, 8, "Blue", "AmphiCar");
av.showInfo();
av.drive();
av.sail();
return 0;
}
```
虚基类继承方式实现:
```cpp
#include <iostream>
using namespace std;
class Vehicle {
protected:
int wheels;
int length;
string color;
int speed;
public:
Vehicle(int w, int l, string c, int s) : wheels(w), length(l), color(c), speed(s) {}
void showInfo() {
cout << "Wheels: " << wheels << endl;
cout << "Length: " << length << endl;
cout << "Color: " << color << endl;
cout << "Speed: " << speed << endl;
}
};
class Ship : virtual public Vehicle {
protected:
int height;
public:
Ship(int l, int h, string c, int s) : Vehicle(0, l, c, s), height(h) {}
void showInfo() {
cout << "Length: " << length << endl;
cout << "Height: " << height << endl;
cout << "Color: " << color << endl;
cout << "Speed: " << speed << endl;
}
};
class AmphibiousVehicle : public Vehicle, public Ship {
private:
string brand;
public:
AmphibiousVehicle(int w, int l, string c, int s, int h, string cc, string b) :
Vehicle(w, l, c, s), Ship(l, h, cc, s), brand(b) {}
void showInfo() {
cout << "Wheels: " << wheels << endl;
cout << "Length: " << length << endl;
cout << "Color: " << color << endl;
cout << "Speed: " << speed << endl;
cout << "Height: " << height << endl;
cout << "Brand: " << brand << endl;
}
void drive() {
cout << "Driving on land." << endl;
}
void sail() {
cout << "Sailing on water." << endl;
}
};
int main() {
AmphibiousVehicle av(4, 6, "Red", 60, 8, "Blue", "AmphiCar");
av.showInfo();
av.drive();
av.sail();
return 0;
}
```
两种实现方式的效果是一样的,都是实现了一个水路两用汽车类。虚基类继承方式相对于普通继承方式,可以避免因为多重继承导致的数据成员重复和不一致的问题,但是虚基类继承的效率相对较低。在实际开发中,需要根据具体情况进行选择。
相关推荐
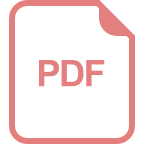
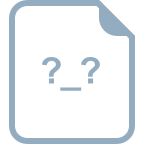
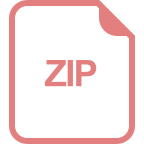














