用c++实现广度优先搜索的代码
时间: 2023-03-31 22:02:43 浏览: 74
可以使用队列来实现广度优先搜索,具体代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTEX_NUM 100 // 最大顶点数
typedef struct ArcNode {
int adjvex; // 邻接点下标
struct ArcNode *next; // 指向下一个邻接点的指针
} ArcNode;
typedef struct VNode {
int data; // 顶点数据
ArcNode *firstarc; // 指向第一个邻接点的指针
} VNode, AdjList[MAX_VERTEX_NUM];
typedef struct {
AdjList vertices; // 邻接表
int vexnum, arcnum; // 顶点数和边数
} ALGraph;
// 初始化邻接表
void InitGraph(ALGraph *G, int n) {
G->vexnum = n;
G->arcnum = ;
for (int i = ; i < n; i++) {
G->vertices[i].data = i;
G->vertices[i].firstarc = NULL;
}
}
// 添加边
void AddEdge(ALGraph *G, int u, int v) {
ArcNode *p = (ArcNode *) malloc(sizeof(ArcNode));
p->adjvex = v;
p->next = G->vertices[u].firstarc;
G->vertices[u].firstarc = p;
G->arcnum++;
}
// 广度优先搜索
void BFS(ALGraph *G, int v) {
int visited[MAX_VERTEX_NUM] = {}; // 标记数组
int queue[MAX_VERTEX_NUM], front = , rear = ; // 队列
visited[v] = 1;
queue[rear++] = v;
while (front < rear) {
int u = queue[front++];
printf("%d ", u);
for (ArcNode *p = G->vertices[u].firstarc; p != NULL; p = p->next) {
int w = p->adjvex;
if (!visited[w]) {
visited[w] = 1;
queue[rear++] = w;
}
}
}
}
int main() {
ALGraph G;
int n, m, u, v;
printf("请输入顶点数和边数:");
scanf("%d%d", &n, &m);
InitGraph(&G, n);
printf("请输入每条边的起点和终点:\n");
for (int i = ; i < m; i++) {
scanf("%d%d", &u, &v);
AddEdge(&G, u, v);
AddEdge(&G, v, u); // 无向图需要加上反向边
}
printf("广度优先搜索结果:");
BFS(&G, );
printf("\n");
return ;
}
```
相关推荐
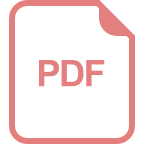
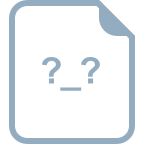














