python将多个列表写入Excel代码
时间: 2024-05-16 20:17:27 浏览: 7
你可以使用Python的openpyxl库将多个列表写入Excel文件中。下面是一个示例代码:
```python
from openpyxl import Workbook
# 创建Excel文件
wb = Workbook()
# 选择工作表
ws = wb.active
# 定义多个列表
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
list3 = [4.5, 6.7, 8.9]
# 写入标题行
ws.append(['列1', '列2', '列3'])
# 写入数据行
for i in range(len(list1)):
ws.append([list1[i], list2[i], list3[i]])
# 保存Excel文件
wb.save('data.xlsx')
```
上面的代码会创建一个名为`data.xlsx`的Excel文件,并在其中写入三个列表的数据。你可以根据需要修改列表的内容和文件名。
相关问题
python把多个列表写入excel
可以使用 Python 的 Pandas 库来将多个列表写入 Excel 文件。下面是一个示例代码:
```python
import pandas as pd
# 定义多个列表
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
list3 = [True, False, True]
# 将多个列表合并成一个数据框
df = pd.DataFrame({'列1': list1, '列2': list2, '列3': list3})
# 将数据框写入 Excel 文件
df.to_excel('output.xlsx', index=False)
```
在这个示例代码中,我们首先定义了三个列表,然后使用 Pandas 的 DataFrame 函数将它们合并成一个数据框。最后,我们使用数据框的 to_excel 方法将数据框写入 Excel 文件。请注意,to_excel 方法的第二个参数 index=False 表示不将行索引写入 Excel 文件中。
python将多个列表依次追加写入excel
可以使用Python中的pandas库来实现将多个列表依次追加写入Excel的操作。具体步骤如下:
1. 导入pandas库。
```python
import pandas as pd
```
2. 定义要写入Excel的文件名和Sheet名。
```python
file_name = 'example.xlsx'
sheet_name = 'Sheet1'
```
3. 读取Excel文件,如果文件不存在,则创建一个新的Excel文件。
```python
try:
df = pd.read_excel(file_name, sheet_name=sheet_name)
except FileNotFoundError:
df = pd.DataFrame()
```
4. 定义要写入Excel的多个列表。
```python
list1 = [1, 2, 3, 4]
list2 = ['A', 'B', 'C', 'D']
list3 = [10.5, 20.5, 30.5, 40.5]
```
5. 将多个列表转换为DataFrame格式。
```python
df1 = pd.DataFrame({'col1': list1})
df2 = pd.DataFrame({'col2': list2})
df3 = pd.DataFrame({'col3': list3})
```
6. 将多个DataFrame按列合并为一个DataFrame。
```python
df_new = pd.concat([df, df1, df2, df3], axis=1)
```
7. 将合并后的DataFrame写入Excel文件。
```python
with pd.ExcelWriter(file_name, mode='a') as writer:
df_new.to_excel(writer, sheet_name=sheet_name, index=False)
```
完整代码如下:
```python
import pandas as pd
file_name = 'example.xlsx'
sheet_name = 'Sheet1'
try:
df = pd.read_excel(file_name, sheet_name=sheet_name)
except FileNotFoundError:
df = pd.DataFrame()
list1 = [1, 2, 3, 4]
list2 = ['A', 'B', 'C', 'D']
list3 = [10.5, 20.5, 30.5, 40.5]
df1 = pd.DataFrame({'col1': list1})
df2 = pd.DataFrame({'col2': list2})
df3 = pd.DataFrame({'col3': list3})
df_new = pd.concat([df, df1, df2, df3], axis=1)
with pd.ExcelWriter(file_name, mode='a') as writer:
df_new.to_excel(writer, sheet_name=sheet_name, index=False)
```
相关推荐
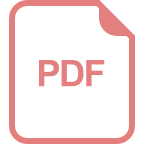
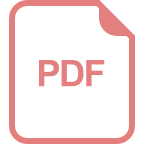
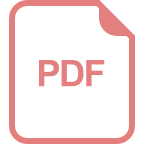












