如何使用c语言使用syscall
时间: 2024-06-17 13:04:08 浏览: 226
在C语言中,使用syscall可以直接调用Linux内核中的系统调用。一般而言,使用syscall需要包含头文件<sys/syscall.h>,并且在代码中使用inline assembly(内联汇编)的方式调用系统调用。
以下是一个简单的示例代码,演示如何使用syscall进行文件操作:
```
#include <stdio.h>
#include <sys/syscall.h>
#include <unistd.h>
int main()
{
char buf[100];
int fd = syscall(SYS_open, "test.txt", O_CREAT | O_WRONLY, 0644);
if (fd < 0) {
perror("open error");
return -1;
}
syscall(SYS_write, fd, "hello syscall\n", 14);
syscall(SYS_lseek, fd, 0, SEEK_SET);
syscall(SYS_read, fd, buf, 14);
printf("%s", buf);
syscall(SYS_close, fd);
return 0;
}
```
在上述代码中,我们使用了三个系统调用:open、write和read,分别用于打开文件、写入数据和读取数据。此外,我们还使用了两个辅助的系统调用:lseek和close,分别用于修改文件读写指针和关闭文件。
相关问题
如何用C语言实现tricore架构的 syscall指令
在TriCore架构中,syscall指令用于实现系统调用。在C语言中,可以通过使用内联汇编来实现syscall指令的调用。以下是一个简单的示例代码:
```
#define __NR_testsyscall 4000
static inline int testsyscall(int arg1, int arg2, int arg3, int arg4)
{
int ret;
__asm__ __volatile__(
"syscall"
: "=a"(ret)
: "0"(__NR_testsyscall), "D"(arg1), "S"(arg2), "d"(arg3), "r"(arg4)
: "memory", "cc"
);
if (ret < 0) {
errno = -ret;
ret = -1;
}
return ret;
}
```
在上面的代码中,__asm__ __volatile__ 是内联汇编的关键字,用于嵌入汇编代码。syscall指令的参数通过寄存器传递,其中__NR_testsyscall是系统调用号,arg1、arg2、arg3和arg4是系统调用的参数。在执行syscall指令后,返回值存储在eax寄存器中。
注意,在TriCore架构中,系统调用的参数和返回值的传递方式可能与其他架构有所不同,具体实现需要根据架构文档进行调整。
syscall_thread main这句话用c语言怎么说
"syscall_thread main" 这句话本身并不是一个完整的语句,它可能是指在操作系统中使用系统调用(syscall)创建线程(thread),并且在主线程(main)中执行其他代码。这个过程可以使用C语言来实现,具体实现方式取决于所使用的操作系统和线程库。一般而言,可以使用类似于pthread_create()函数的库函数来创建线程,然后在主函数中执行其他代码。例如,在Linux系统中,可以使用系统调用clone()来创建线程,然后使用类似于以下的代码来实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/syscall.h>
#include <sys/types.h>
#define gettid() syscall(SYS_gettid)
void *thread_func(void *arg) {
printf("In thread_func, thread ID = %ld\n", gettid());
return NULL;
}
int main() {
pthread_t tid;
printf("In main, thread ID = %ld\n", gettid());
if (pthread_create(&tid, NULL, thread_func, NULL) != 0) {
perror("pthread_create");
exit(EXIT_FAILURE);
}
pthread_join(tid, NULL);
return 0;
}
```
这个代码片段中,主函数中使用gettid()获取主线程的线程ID,然后在创建新线程时,使用pthread_create()函数创建一个新线程,并将其执行函数指定为thread_func(),然后使用pthread_join()函数等待新线程结束。在thread_func()函数中,使用gettid()获取当前线程的线程ID,并进行输出。
阅读全文
相关推荐
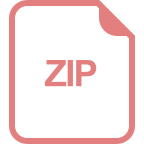
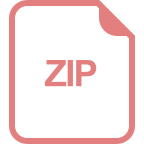
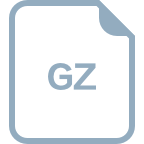
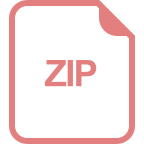
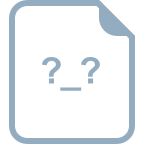
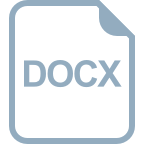
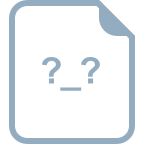
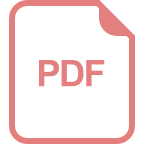
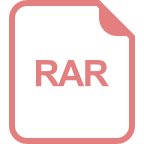
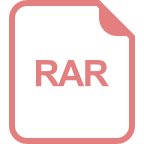
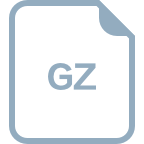
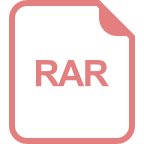
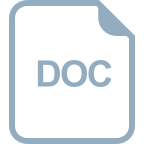

