使用链表实现一元多项式的输入输出C++
时间: 2024-10-14 17:13:44 浏览: 30
链表可以用于表示一元多项式,这是因为链表的数据结构非常适合存储一系列元素,每个元素通常包含系数和变量指数。在C++中,你可以创建一个`Node`类来代表多项式的每一项:
```cpp
class Node {
public:
double coefficient; // 系数
int exponent; // 指数
// 构造函数
Node(double c = 0, int e = 0) : coefficient(c), exponent(e) {}
// 为了形成链表,我们可以添加一个指向下一个节点的指针
Node* next = nullptr;
};
```
然后,你可以创建一个`Polynomial`类,它维护一个头结点,并提供操作函数:
```cpp
class Polynomial {
private:
Node* head;
public:
Polynomial() { head = nullptr; }
// 添加项到多项式
void addTerm(double c, int e) {
if (!head) {
head = new Node(c, e);
} else {
Node* current = head;
while (current->next) {
current = current->next;
}
current->next = new Node(c, e);
}
}
// 输出多项式(默认按升序)
void print() const {
Node* temp = head;
while (temp) {
std::cout << temp->coefficient << "x^" << temp->exponent << " + ";
temp = temp->next;
}
std::cout << "0" << std::endl; // 结束字符串并打印常数项
}
// 输入多项式,例如 "2x^2 + 5x - 7"
void input(const std::string& str) {
std::istringstream iss(str);
std::string term;
while (iss >> term) {
double coef;
int exp;
size_t pos = term.find("x");
if (pos != std::string::npos) {
coef = std::stod(term.substr(0, pos));
exp = std::stoi(term.substr(pos + 1));
addTerm(coef, exp);
}
}
}
};
```
使用这个类,你可以创建一个多项式对象,然后输入和输出多项式:
```cpp
int main() {
Polynomial poly;
poly.input("2x^2 + 5x - 7"); // 输入多项式
poly.print(); // 输出多项式
return 0;
}
```
阅读全文
相关推荐
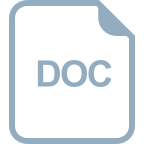
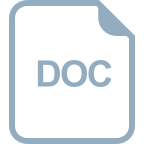
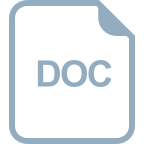
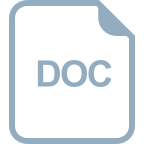





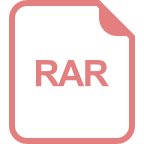
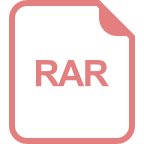
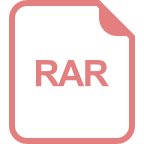
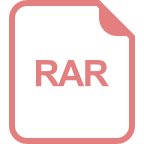
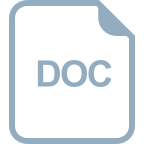
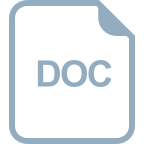
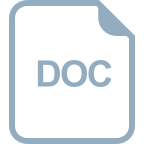
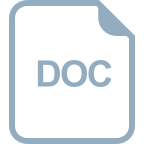

