startPoint.setX(inputX1);中括号处应该填写什么
时间: 2024-12-20 11:21:36 浏览: 14
`setX()` 和 `setY()` 是坐标点类(如 `QPointF`, `QPoint`, 或 `QVector2D`)的成员函数,它们用于设置点的位置。在这个上下文中,`inputX1` 和 `inputY1` 应该是你程序中存储的两个坐标值,分别代表直线起点的横纵坐标。
所以 `startPoint.setX(inputX1)` 中,`inputX1` 是一个变量名,它通常表示用户输入的第一个x坐标或者是预先设定的一个初始值,用来确定直线的开始位置。如果你正在处理用户输入,可能是从键盘、鼠标点击或者其他输入设备获取的。例如,如果是在窗口上监听鼠标点击事件,那么这个值可能是点击事件的位置.x属性。
完整的例子可能是这样的:
```cpp
QPointF startPoint; // 创建一个坐标点对象
int inputX1 = getUserInputX(); // 获取用户输入的x坐标
int inputY1 = getUserInputY(); // 获取用户输入的y坐标
// 然后设置起点坐标
startPoint.setX(inputX1);
startPoint.setY(inputY1);
```
相关问题
registerEvent () { let endPoint = cc.v2(0,0) let startPoint = cc.v2(0,0) this.node.on(cc.Node.EventType.TOUCH_MOVE, (e) => { startPoint = e.getStartLocation(); endPoint = e.getLocation(); const distance = startPoint.sub(endPoint).mag(); if (distance <= 300) { this.draw.clear(); this.draw.moveTo(startPoint.x, startPoint.y); this.draw.lineTo(e.getLocationX(), e.getLocationY()); this.draw.stroke(); } }, this); this.node.on(cc.Node.EventType.TOUCH_END, (e) => { this.draw.clear(); let lineStart = startPoint.clone(); // 保存 300 线段的起点 let lineEnd = endPoint.clone(); // 保存 300 线段的终点 this.cut(lineStart, lineEnd); // 在 cut() 方法中处理 300 线段 }, this); } cut(pOne,pTwo){ let ropeJoints: cc.RopeJoint[] = [] if(this.chapterOne.active === true){ //ropeJoints = cc.director.getScene().getComponentsInChildren(cc.RopeJoint); ropeJoints =this.chapterOne.getComponentsInChildren(cc.RopeJoint); } else if(this.chapterTwo.active === true) { //ropeJoints = cc.director.getScene().getComponentsInChildren(cc.RopeJoint); ropeJoints =this.chapterTwo.getComponentsInChildren(cc.RopeJoint); } ropeJoints.forEach((item) => { if(item.node.width ==10&&item.node.height==10){ let lineP1 = pOne; // 线段起点 let lineP2 = pTwo; // 线段终点 let rectPoint = cc.v2(item.node.x-5, item.node.y-5) let rectWorldPoint = item.node.convertToWorldSpaceAR(rectPoint) let rect = cc.rect(rectWorldPoint.x,rectWorldPoint.y, 20, 20); // 矩形,左下角坐标为(5,5),宽高为10 let isIntersect = cc.Intersection.lineRect(lineP1, lineP2, rect); console.log(isIntersect); // 输出 true 或 false if(isIntersect== true){ item.node.parent.active = false } } }); }
这段代码实现了一个简单的画线和切割功能。当手指在屏幕上滑动时,会在画布上绘制一条直线;当手指离开屏幕时,会根据画线的起点和终点计算出一条线段,并将该线段和所有的 `cc.RopeJoint` 组件进行碰撞检测,如果检测到碰撞则将相应的节点隐藏。
其中,`registerEvent()` 方法用于注册触摸事件,`cut()` 方法用于进行碰撞检测。
需要注意的是,这段代码仅适用于场景中只有 `cc.RopeJoint` 组件的情况,如果场景中还有其他类型的组件,需要修改代码以区分不同类型的组件。另外,代码中的一些变量和方法没有给出定义,需要根据具体情况进行修改。
private void MyControl_MouseMove(object sender, MouseEventArgs e) { if(sender is UIElement uIElement) { if (isDragging) { Point newPoint = e.GetPosition(canvas); double deltaX = newPoint.X - startPoint.X; double deltaY = newPoint.Y - startPoint.Y; Canvas.SetLeft(uIElement, Canvas.GetLeft(uIElement) + deltaX); Canvas.SetTop(uIElement, Canvas.GetTop(uIElement) + deltaY); startPoint = newPoint; } } } private void MyControl_MouseDown(object sender, MouseButtonEventArgs e) { if (sender is UIElement uIElement) { isDragging = true; startPoint = e.GetPosition(canvas); uIElement.CaptureMouse(); } } private void MyControl_MouseUp(object sender, MouseButtonEventArgs e) { if (sender is UIElement uIElement) { isDragging = false; uIElement.ReleaseMouseCapture(); } }令移动的控件不超过Canvas的边界
可以在 `MyControl_MouseMove` 方法中添加一些代码来检查移动的控件是否超过了 `Canvas` 的边界。具体实现方法如下:
```
private void MyControl_MouseMove(object sender, MouseEventArgs e)
{
if (sender is UIElement uIElement)
{
if (isDragging)
{
Point newPoint = e.GetPosition(canvas);
double deltaX = newPoint.X - startPoint.X;
double deltaY = newPoint.Y - startPoint.Y;
// 计算控件新的位置
double newX = Canvas.GetLeft(uIElement) + deltaX;
double newY = Canvas.GetTop(uIElement) + deltaY;
// 检查控件是否超过 Canvas 的边界
if (newX < 0)
{
newX = 0;
}
else if (newX + uIElement.ActualWidth > canvas.ActualWidth)
{
newX = canvas.ActualWidth - uIElement.ActualWidth;
}
if (newY < 0)
{
newY = 0;
}
else if (newY + uIElement.ActualHeight > canvas.ActualHeight)
{
newY = canvas.ActualHeight - uIElement.ActualHeight;
}
Canvas.SetLeft(uIElement, newX);
Canvas.SetTop(uIElement, newY);
startPoint = newPoint;
}
}
}
```
在上述代码中,我们首先计算出控件的新位置 `newX` 和 `newY`,然后通过一些判断来确保控件不超过 `Canvas` 的边界。如果控件超过了边界,则将其位置设置为边界的位置。最后,我们调用 `Canvas.SetLeft` 和 `Canvas.SetTop` 方法来更新控件的位置。
阅读全文
相关推荐
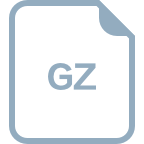
















