以下代码 ,拖动功能正常,但是在用两根手指进行缩放和旋转时闪退,请您完善 。public boolean onTouchEvent(MotionEvent event) { switch (event.getAction() & MotionEvent.ACTION_MASK) { case MotionEvent.ACTION_DOWN: // 手指压下屏幕 mode = MODE.DRAG; // 查找被点击的图片 int index = event.getActionIndex(); float x = event.getX(index); float y = event.getY(index); CustomBitmap clickedBitmap = findClickedBitmap(x, y); if (clickedBitmap != null) { // 切换操作对象 _bitmaps.remove(clickedBitmap); _bitmaps.add(clickedBitmap); // 记录ImageView当前的移动位置 currentMatrix.set(clickedBitmap.matrix); clickedBitmap.matrix.set(currentMatrix); clickedBitmap.startPoint.set(x, y); _curCustomBitmap = clickedBitmap; } postInvalidate(); break; case MotionEvent.ACTION_POINTER_DOWN: // 当屏幕上还有触点(手指),再有一个手指压下屏幕 mode = MODE.ZOOM; // 记录位图的旋转角度和缩放倍数 if (_curCustomBitmap == null) { return true; } _curCustomBitmap.oldRotation = rotation(event); _curCustomBitmap.startDis = distance(event); if (_curCustomBitmap.startDis > 10f) { // 获取缩放中心点的坐标 float x1 = event.getX(0); float y1 = event.getY(0); float x2 = event.getX(1); float y2 = event.getY(1); _curCustomBitmap.midPoint.set((x1 + x2) / 2, (y1 + y2) / 2); // 记录ImageView当前的缩放倍数 currentMatrix.set(_curCustomBitmap.matrix); } break; case MotionEvent.ACTION_MOVE: // 手指在屏幕移动,该事件会不断地触发 if (mode == MODE.DRAG) { // 移动图片 if (_curCustomBitmap == null) { return true; } float dx = event.getX() - _curCustomBitmap.startPoint.x; float dy = event.getY() - _curCustomBitmap.startPoint.y; _curCustomBitmap.matrix.set(currentMatrix); _curCustomBitmap.matrix.postTranslate(dx, dy); } else if (mode == MODE.ZOOM) { // 缩放和旋转图片 if (_curCustomBitmap == null) { return true; } float endDis = distance(event); float rotation = rotation(event) - _curCustomBitmap.oldRotation; if (endDis > 10f) { float scale = endDis / _curCustomBitmap.startDis; _curCustomBitmap.matrix.set(currentMatrix); _curCustomBitmap.matrix.postScale(scale, scale, _curCustomBitmap.midPoint.x, _curCustomBitmap.midPoint.y); _curCustomBitmap.matrix.postRotate(rotation, _curCustomBitmap.midPoint.x, _curCustomBitmap.midPoint.y); } } break; case MotionEvent.ACTION_POINTER_UP: // 有手指离开屏幕,但屏幕还有触点(手指) mode = MODE.NONE; break; } invalidate(); return true; } // 查找被点击的图片 private CustomBitmap findClickedBitmap(float x, float y) { for (CustomBitmap bitmap : _bitmaps) { float[] values = new float[9]; bitmap.matrix.getValues(values); float globalX = values[Matrix.MTRANS_X]; float globalY = values[Matrix.MTRANS_Y]; float width = values[Matrix.MSCALE_X] * bitmap.getBitmap().getWidth(); float height = values[Matrix.MSCALE_Y] * bitmap.getBitmap().getHeight(); RectF rect = new RectF(globalX, globalY, globalX + width, globalY + height); if (rect.contains(x, y)) { return bitmap; } } return null; } // 计算两点之间的距离 private float distance(MotionEvent event) { float x1 = event.getX(0); float y1 = event.getY(0); float x2 = event.getX(1); float y2 = event.getY(1); return (float) Math.sqrt((x1 - x2) * (x1 - x2) + (y1 - y2) * (y1 - y2)); } // 计算两点之间的旋转角度 private float rotation(MotionEvent event) { float x1 = event.getX(0); float y1 = event.getY(0); float x2 = event.getX(1); float y2 = event.getY(1); double radians = Math.atan2(y2 - y1, x2 - x1); return (float) Math.toDegrees(radians); }
时间: 2024-03-05 21:50:36 浏览: 19
在处理 MotionEvent.ACTION_POINTER_UP 事件时,需要判断当前屏幕上是否还有触点(手指),如果没有触点了,需要将 mode 设置为 MODE.NONE ,否则会导致下一次触摸事件处理时出现问题。另外,在处理 MotionEvent.ACTION_MOVE 事件时,需要判断当前的 mode 是 MODE.DRAG 还是 MODE.ZOOM,只有在 mode 为 MODE.DRAG 时才需要移动图片,而在 mode 为 MODE.ZOOM 时才需要缩放和旋转图片。完善后的代码如下所示:
```
public boolean onTouchEvent(MotionEvent event) {
switch (event.getAction() & MotionEvent.ACTION_MASK) {
case MotionEvent.ACTION_DOWN:
// 手指压下屏幕
mode = MODE.DRAG;
// 查找被点击的图片
int index = event.getActionIndex();
float x = event.getX(index);
float y = event.getY(index);
CustomBitmap clickedBitmap = findClickedBitmap(x, y);
if (clickedBitmap != null) {
// 切换操作对象
_bitmaps.remove(clickedBitmap);
_bitmaps.add(clickedBitmap);
// 记录ImageView当前的移动位置
currentMatrix.set(clickedBitmap.matrix);
clickedBitmap.matrix.set(currentMatrix);
clickedBitmap.startPoint.set(x, y);
_curCustomBitmap = clickedBitmap;
}
postInvalidate();
break;
case MotionEvent.ACTION_POINTER_DOWN:
// 当屏幕上还有触点(手指),再有一个手指压下屏幕
mode = MODE.ZOOM;
// 记录位图的旋转角度和缩放倍数
if (_curCustomBitmap == null) {
return true;
}
_curCustomBitmap.oldRotation = rotation(event);
_curCustomBitmap.startDis = distance(event);
if (_curCustomBitmap.startDis > 10f) {
// 获取缩放中心点的坐标
float x1 = event.getX(0);
float y1 = event.getY(0);
float x2 = event.getX(1);
float y2 = event.getY(1);
_curCustomBitmap.midPoint.set((x1 + x2) / 2, (y1 + y2) / 2);
// 记录ImageView当前的缩放倍数
currentMatrix.set(_curCustomBitmap.matrix);
}
break;
case MotionEvent.ACTION_MOVE:
// 手指在屏幕移动,该事件会不断地触发
if (mode == MODE.DRAG) {
// 移动图片
if (_curCustomBitmap == null) {
return true;
}
float dx = event.getX() - _curCustomBitmap.startPoint.x;
float dy = event.getY() - _curCustomBitmap.startPoint.y;
_curCustomBitmap.matrix.set(currentMatrix);
_curCustomBitmap.matrix.postTranslate(dx, dy);
} else if (mode == MODE.ZOOM) {
// 缩放和旋转图片
if (_curCustomBitmap == null) {
return true;
}
float endDis = distance(event);
float rotation = rotation(event) - _curCustomBitmap.oldRotation;
if (endDis > 10f) {
float scale = endDis / _curCustomBitmap.startDis;
_curCustomBitmap.matrix.set(currentMatrix);
_curCustomBitmap.matrix.postScale(scale, scale, _curCustomBitmap.midPoint.x, _curCustomBitmap.midPoint.y);
_curCustomBitmap.matrix.postRotate(rotation, _curCustomBitmap.midPoint.x, _curCustomBitmap.midPoint.y);
}
}
break;
case MotionEvent.ACTION_POINTER_UP:
// 有手指离开屏幕,但屏幕还有触点(手指)
if (event.getPointerCount() > 1) {
mode = MODE.ZOOM;
} else {
mode = MODE.NONE;
}
break;
}
invalidate();
return true;
}
```
相关推荐
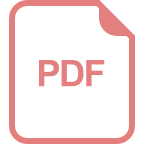
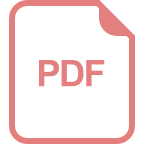
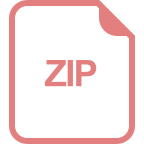














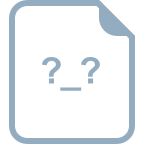