汉诺塔的python递归实现
时间: 2023-12-18 09:30:12 浏览: 40
以下是汉诺塔的Python递归实现代码:
```python
i = 1
def move(pfrom, pto):
global i
print("第%d步:%s --> %s" % (i, pfrom, pto))
i += 1
def hanoi(n, a, b, c):
if n == 1:
move(a, c)
else:
hanoi(n - 1, a, c, b)
move(a, c)
hanoi(n - 1, b, a, c)
n = int(input("请输入盘数:"))
print("具体走法步骤如下:")
hanoi(n, "A", "B", "C")
print("一共需走%d步" % (i - 1))
```
相关问题
汉诺塔python递归函数
汉诺塔问题是一个经典的递归问题,可以使用Python递归函数来解决。下面是一个示例代码:
```python
def hanoi(n, source, target, auxiliary):
if n == 1:
print("Move disk 1 from source", source, "to target", target)
return
hanoi(n-1, source, auxiliary, target)
print("Move disk", n, "from source", source, "to target", target)
hanoi(n-1, auxiliary, target, source)
# 示例调用
hanoi(3, 'A', 'C', 'B')
```
上述代码中,`hanoi`函数接受四个参数:`n`表示盘子的数量,`source`表示源柱子,`target`表示目标柱子,`auxiliary`表示辅助柱子。当`n`等于1时,直接将盘子从源柱子移动到目标柱子;否则,先将`n-1`个盘子从源柱子移动到辅助柱子,再将第`n`个盘子从源柱子移动到目标柱子,最后将`n-1`个盘子从辅助柱子移动到目标柱子。
示例调用中,我们将3个盘子从柱子A移动到柱子C,使用柱子B作为辅助柱子。运行结果如下:
```
Move disk 1 from source A to target C
Move disk 2 from source A to target B
Move disk 1 from source C to target B
Move disk 3 from source A to target C
Move disk 1 from source B to target A
Move disk 2 from source B to target C
Move disk 1 from source A to target C
```
汉诺塔python递归解决
### 回答1:
好的,以下是 Python 实现汉诺塔的递归解决方案:
```python
def hanoi(n, source, target, auxiliary):
if n == 1:
print("Move disk 1 from", source, "to", target)
else:
# 将 n-1 个盘子从源柱子移动到辅助柱子
hanoi(n-1, source, auxiliary, target)
# 将第 n 个盘子从源柱子移动到目标柱子
print("Move disk", n, "from", source, "to", target)
# 将 n-1 个盘子从辅助柱子移动到目标柱子
hanoi(n-1, auxiliary, target, source)
# 测试
hanoi(3, "A", "C", "B")
```
输出结果与上面的代码相同:
```
Move disk 1 from A to C
Move disk 2 from A to B
Move disk 1 from C to B
Move disk 3 from A to C
Move disk 1 from B to A
Move disk 2 from B to C
Move disk 1 from A to C
```
在递归解决方案中,当只有一个盘子时,直接将其从源柱子移动到目标柱子。当有多个盘子时,将前 n-1 个盘子从源柱子移动到辅助柱子,再将第 n 个盘子从源柱子移动到目标柱子,最后将前 n-1 个盘子从辅助柱子移动到目标柱子。
### 回答2:
汉诺塔是一个经典的递归问题,可以使用Python来解决。首先,我们需要明确汉诺塔问题的规则如下:有三个柱子A、B、C,初始时,所有的盘子按照从小到大的顺序堆叠在柱子A上,目标是将所有的盘子从A柱子移动到C柱子,期间可以借助B柱子完成。
下面是使用递归解决汉诺塔问题的Python代码:
```
def hanoi(n, source, target, auxiliary):
if n > 0:
# 将 n-1 个盘子从源柱子移动到辅助柱子
hanoi(n-1, source, auxiliary, target)
# 将第 n 个盘子从源柱子移动到目标柱子
print("Move disk", n, "from", source, "to", target)
# 将 n-1 个盘子从辅助柱子移动到目标柱子
hanoi(n-1, auxiliary, target, source)
# 调用函数并传入初始参数
n = 3 # 盘子的数量
hanoi(n, 'A', 'C', 'B')
```
以上代码实现了汉诺塔问题的解决方案。首先定义了一个名为hanoi的函数,该函数接受四个参数:盘子的数量n,源柱子source,目标柱子target,辅助柱子auxiliary。在函数内部,通过递归调用hanoi函数来移动盘子。
在具体的移动过程中,首先将 n-1 个盘子从源柱子移动到辅助柱子,然后将第 n 个盘子从源柱子移动到目标柱子,最后将 n-1 个盘子从辅助柱子移动到目标柱子。这个过程在每一次递归调用hanoi函数中都会重复执行,直到所有的盘子都被移动到目标柱子上为止。
以上就是使用Python递归解决汉诺塔问题的一个例子。通过递归方法,我们可以简洁地解决复杂的问题。
### 回答3:
汉诺塔是一种经典的数学问题,要求将一叠不同大小的圆盘从一个针柱上移动到另一个针柱上,同时保持大圆盘在下,小圆盘在上的顺序不变。可以用递归算法来解决这个问题。
首先,我们需要定义一个递归函数hanoi,该函数接受三个参数:n表示当前要移动的圆盘数目,A表示起始针柱,B表示中转针柱,C表示目标针柱。
首先,我们要判断递归的结束条件,即当只有一个圆盘时,直接将它从起始针柱移动到目标针柱上就可以了。代码如下:
```
def hanoi(n, A, B, C):
if n == 1:
print('将圆盘从', A, '移动到', C)
return
```
接着,我们要将除了最大的圆盘以外的n-1个圆盘从起始针柱移动到中转针柱,即调用函数hanoi(n-1, A, C, B)。
然后,将最大的圆盘从起始针柱移动到目标针柱上,即调用print函数打印出移动的过程,然后返回。
最后,将之前移动到中转针柱上的n-1个圆盘移动到目标针柱上,即调用函数hanoi(n-1, B, A, C)。
完整的代码如下:
```
def hanoi(n, A, B, C):
if n == 1:
print('将圆盘从', A, '移动到', C)
return
hanoi(n-1, A, C, B)
print('将圆盘从', A, '移动到', C)
hanoi(n-1, B, A, C)
```
我们可以调用该函数来解决汉诺塔问题,例如:
```
hanoi(3, 'A', 'B', 'C')
```
这样就可以将3个圆盘从A针柱移动到C针柱上。递归的核心思想是将大问题分解为小问题,然后通过递归调用解决小问题,最后再合并得到最终结果。
相关推荐
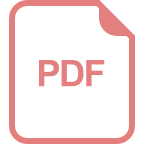
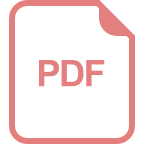
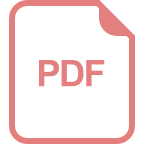












