dfs bfs java
时间: 2023-08-29 07:13:42 浏览: 51
DFS (Depth First Search) and BFS (Breadth First Search) are two popular graph traversal algorithms used in computer science. Here is an example implementation of both algorithms in Java:
DFS:
```
import java.util.*;
public class DFS {
private int V; // number of vertices
private LinkedList<Integer> adj[]; // adjacency list
DFS(int v) {
V = v;
adj = new LinkedList[v];
for (int i=0; i<v; ++i)
adj[i] = new LinkedList();
}
// add an edge to the graph
void addEdge(int v, int w) {
adj[v].add(w);
}
// depth first search from a given node
void DFSUtil(int v, boolean visited[]) {
visited[v] = true;
System.out.print(v + " ");
Iterator<Integer> i = adj[v].listIterator();
while (i.hasNext()) {
int n = i.next();
if (!visited[n])
DFSUtil(n, visited);
}
}
// depth first search traversal
void DFS(int v) {
boolean visited[] = new boolean[V];
DFSUtil(v, visited);
}
public static void main(String args[]) {
DFS g = new DFS(4);
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 2);
g.addEdge(2, 0);
g.addEdge(2, 3);
g.addEdge(3, 3);
System.out.println("Depth First Traversal (starting from vertex 2):");
g.DFS(2);
}
}
```
BFS:
```
import java.util.*;
public class BFS {
private int V; // number of vertices
private LinkedList<Integer> adj[]; // adjacency list
BFS(int v) {
V = v;
adj = new LinkedList[v];
for (int i=0; i<v; ++i)
adj[i] = new LinkedList();
}
// add an edge to the graph
void addEdge(int v, int w) {
adj[v].add(w);
}
// breadth first search traversal
void BFS(int s) {
boolean visited[] = new boolean[V];
LinkedList<Integer> queue = new LinkedList<Integer>();
visited[s] = true;
queue.add(s);
while (queue.size() != 0) {
s = queue.poll();
System.out.print(s + " ");
Iterator<Integer> i = adj[s].listIterator();
while (i.hasNext()) {
int n = i.next();
if (!visited[n]) {
visited[n] = true;
queue.add(n);
}
}
}
}
public static void main(String args[]) {
BFS g = new BFS(4);
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 2);
g.addEdge(2, 0);
g.addEdge(2, 3);
g.addEdge(3, 3);
System.out.println("Breadth First Traversal (starting from vertex 2):");
g.BFS(2);
}
}
```
相关推荐
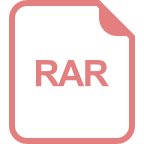
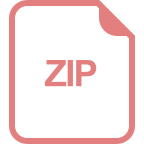














