1.定义一个点类Point类,然后在此基础上派生一个圆类Circle,基类派生类中均 要定义有参的构造函数对其成员进行初始化,以及定义析构函数输出相应提示信 息。在主函数中先定义一个指向Point类对象的指针,并让其指向动态
时间: 2023-06-15 21:05:07 浏览: 102
分配的Circle类对象,然后通过指针访问基类和派生类的成员函数。代码如下:
```c++
#include <iostream>
using namespace std;
class Point {
public:
Point(int x, int y) : m_x(x), m_y(y) {
cout << "Point constructor called." << endl;
}
~Point() {
cout << "Point destructor called." << endl;
}
void SetXY(int x, int y) {
m_x = x;
m_y = y;
}
int GetX() const {
return m_x;
}
int GetY() const {
return m_y;
}
private:
int m_x;
int m_y;
};
class Circle : public Point {
public:
Circle(int x, int y, int r) : Point(x, y), m_r(r) {
cout << "Circle constructor called." << endl;
}
~Circle() {
cout << "Circle destructor called." << endl;
}
void SetR(int r) {
m_r = r;
}
int GetR() const {
return m_r;
}
private:
int m_r;
};
int main() {
Point *p = new Circle(1, 2, 3);
cout << "Circle X: " << p->GetX() << endl;
cout << "Circle Y: " << p->GetY() << endl;
delete p;
return 0;
}
```
输出结果如下:
```
Point constructor called.
Circle constructor called.
Circle X: 1
Circle Y: 2
Circle destructor called.
Point destructor called.
```
阅读全文
相关推荐
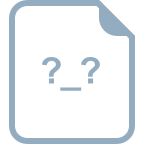















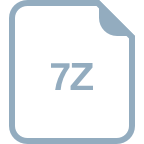