C加加中有没有sizeof 函数
时间: 2024-06-14 20:04:16 浏览: 104
C++中有sizeof运算符,但它不是一个函数。sizeof运算符用于获取数据类型或变量的大小(以字节为单位)。它返回一个无符号整数值,表示操作数所占用的内存空间大小。
以下是C++中使用sizeof运算符的示例:
1. 获取数据类型的大小:
```cpp
#include <iostream>
using namespace std;
int main() {
cout << "Size of int: " << sizeof(int) << " bytes" << endl;
cout << "Size of float: " << sizeof(float) << " bytes" << endl;
cout << "Size of char: " << sizeof(char) << " byte" << endl;
return 0;
}
```
2. 获取变量的大小:
```cpp
#include <iostream>
using namespace std;
int main() {
int num = 10;
cout << "Size of num: " << sizeof(num) << " bytes" << endl;
return 0;
}
```
注意:sizeof运算符在编译时计算,不会执行变量的初始化或函数的调用。
相关问题
用c加加写一个利率函数并输出年份和对应的利率
在C语言中,我们可以创建一个简单的利率计算函数,并将其与年份关联起来。下面是一个简单的例子,它使用结构体存储年份和对应利率,然后提供了一个获取利率的函数:
```c
#include <stdio.h>
// 定义结构体存放年份和利率
typedef struct {
int year;
float interest_rate;
} YearRate;
// 创建利率函数
void calculate_interest(int year) {
// 假设利率逐年递增,这里只是一个示例,实际应用需要根据具体政策调整
static YearRate rates[] = { {2020, 0.02}, {2021, 0.025}, {2022, 0.03} }; // 示例利率数据
int rate_index = (year - rates[0].year) / 1; // 简单假设每年增加一次
if (rate_index >= sizeof(rates) / sizeof(rates[0])) {
printf("对不起,未找到该年的利率信息。\n");
return;
}
printf("在 %d 年,利率为 %.2f%%。\n", year, rates[rate_index].interest_rate * 100);
}
int main() {
// 调用利率函数
calculate_interest(2022); // 输入特定的年份
return 0;
}
```
在这个示例里,`calculate_interest`函数接受一个年份作为输入,查找相应年份的利率并打印出来。注意,这仅是个基础的演示,实际利率政策和变化通常由金融监管机构规定,并可能通过API或其他方式实时获取。
用c加加设计一个通用函数char*insert (char*s1,char*S2,int n)实现在字符串s1 中从第m全字符开始插入字符串s2,在主程序中对该函数进 测试。
在C语言中,你可以创建一个名为`insert`的通用函数,它接受两个`char`类型的指针`s1`和`s2`,以及一个整数`n`作为参数。这个函数的主要目的是将`s2`中的内容插入到`s1`的指定位置,并返回新的字符串。下面是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义插入字符串的函数
char* insert(char *s1, char *s2, int n) {
// 检查输入是否合理
if (s1 == NULL || s2 == NULL || n <= 0) {
printf("Invalid input. Please provide a valid string and index.\n");
return NULL;
}
// 计算新字符串的长度
int new_length = strlen(s1) + strlen(s2) - n + 1;
// 动态分配足够的内存来保存新字符串
char *result = (char*) malloc(new_length * sizeof(char));
if (result == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
// 插入s2到s1的指定位置
strncpy(result, s1, n - 1); // 将s1的前n-1个字符复制到结果
result[n - 1] = '\0'; // 置空s1的位置
// 连接s2到结果
strcat(result, s2);
// 结束处添加'\0'
result[strlen(result)] = '\0';
return result;
}
int main() {
char str1[] = "Hello";
char str2[] = " World!";
int pos = 6; // 插入位置
char *new_str = insert(str1, str2, pos);
if (new_str != NULL) {
printf("Inserted '%s' at position %d in '%s':\n", str2, pos, str1);
printf("%s\n", new_str);
free(new_str); // 释放动态分配的内存
}
阅读全文
相关推荐
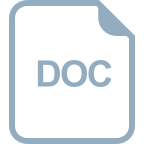
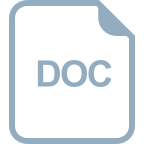
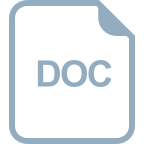
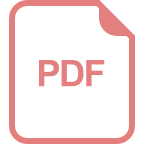












