In [107]: from sklearn.linear_model import LogisticRegressionCV In [108]: model_cv = LogisticRegressionCV(10) In [109]: model_cv.fit(X_train, y_train) Out[109]: LogisticRegressionCV(Cs=10, class_weight=None, cv=None, dual=False, fit_intercept=True, intercept_scaling=1.0, max_iter=100, multi_class='ovr', n_jobs=1, penalty='l2', random_state=None, refit=True, scoring=None, solver='lbfgs', tol=0.0001, verbose=0)
时间: 2024-03-31 07:34:19 浏览: 87
这是一个使用scikit-learn库中的LogisticRegressionCV模型进行交叉验证的Python代码段。首先,从sklearn.linear_model模块中导入LogisticRegressionCV类。接着,创建一个LogisticRegressionCV对象model_cv,其中指定了参数Cs=10,表示将在10个不同的正则化强度下执行交叉验证。然后,使用fit方法将模型拟合到训练数据集(X_train, y_train)上。最后,输出模型对象,其中包含了模型参数的详细信息和交叉验证结果等信息。
相关问题
import lightgbm as lgb from lightgbm import LGBMClassifier from sklearn.metrics import accuracy_score from sklearn.model_selection import cross_val_score from sklearn.model_selection import GridSearchCV # 定义xgboost模型 lgb_model = lgb.LGBMClassifier() # 设置参数空间 params = { 'boosting_type': 'gbdt', #'boosting_type': 'dart', 'objective': 'multiclass', 'metric': 'multi_logloss', 'min_child_weight': 1.5, 'num_leaves': 2**5, 'lambda_l2': 10, 'subsample': 0.7, 'colsample_bytree': 0.7, 'learning_rate': 0.03, 'seed': 2017, "num_class": 2, 'silent': True, } # 输出最优参数 print('Best Parameter:', params) # 使用最优参数构建新的xgboost模型 lgb_model = lgb.LGBMClassifier(**params) # 使用训练集对新模型进行拟合 # X_train = np.array(X_train) # pdb.set_trace() lgb_model.fit(X_train, y_train) # 使用新模型进行预测 y_pred = lgb_model.predict(X_test)改为svm模型
好的,那么你需要将导入的库改为sklearn中的SVM库,代码如下:
```python
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
from sklearn.model_selection import cross_val_score
from sklearn.model_selection import GridSearchCV
# 定义svm模型
svm_model = SVC()
# 设置参数空间
params = {
'kernel': ['linear', 'rbf', 'poly', 'sigmoid'],
'C': [0.1, 1, 10, 100],
'gamma': ['scale', 'auto', 0.1, 1, 10],
'decision_function_shape': ['ovo', 'ovr'],
'random_state': [2017]
}
# 输出最优参数
print('Best Parameter:', params)
# 使用GridSearchCV进行参数调优
svm_model = GridSearchCV(svm_model, params, cv=5)
# 使用训练集对新模型进行拟合
svm_model.fit(X_train, y_train)
# 使用新模型进行预测
y_pred = svm_model.predict(X_test)
```
注意,SVM模型的参数设置有很多,你需要根据你的数据集和问题场景来进行调整。在上面的代码中,我使用了GridSearchCV进行了参数调优,你可以根据需要进行修改。
import numpy as np import xlrd import matplotlib.pyplot as plt from sklearn.feature_selection import RFE from sklearn.ensemble import RandomForestClassifier from sklearn.svm import SVC from sklearn.linear_model import LogisticRegression from sklearn.model_selection import cross_val_score def excel2m(path):#读excel数据转为矩阵函数 data = xlrd.open_workbook(path) table = data.sheets()[0] # 获取excel中第一个sheet表 nrows = table.nrows # 行数 ncols = table.ncols # 列数 datamatrix = np.zeros((nrows, ncols)) for x in range(ncols): cols = table.col_values(x) cols1 = np.matrix(cols) # 把list转换为矩阵进行矩阵操作 datamatrix[:, x] = cols1 # 把数据进行存储 return datamatrix x=excel2m("factors.xlsx") x=np.matrix(x) y=excel2m("RON.xlsx") y=np.matrix(y) rfc=RandomForestClassifier(n_estimators=10,random_state=0) score=[] for i in range(1,200,10): rfe = RFE(estimator=rfc, n_features_to_select=i, step=10).fit(x, y.astype('int')) rfe.support_.sum() rfe.ranking_ x_wrapper=rfe.transform(x) once=cross_val_score(rfc,x_wrapper,y.astype('int'),cv=5).mean() score.append(once) plt.figure(figsize=[20,5]) plt.plot(range(1,200,10),score) plt.xticks(range(1,200,10)) plt.show() np.savetxt('score.csv', score, delimiter = ',') # 确定选择特征数量后,看各个特征得分排名 # 每个特征的得分排名,特征得分越低(1最好),表示特征越好 #print(rfe.ranking_) #np.savetxt('ranking.csv', rfe.ranking_, delimiter = ',') # 每次交叉迭代各个特征得分 #print(rfe.grid_scores_) #np.savetxt('grid_scores.csv', rfe.grid_scores_, delimiter = ',')
这段代码主要实现的功能是特征筛选,其中使用了随机森林分类器(RandomForestClassifier)和递归特征消除算法(RFE),以提高模型的准确性和降低过拟合的风险。
具体流程为:首先将读取的excel数据转换为矩阵形式,然后通过循环调整特征数量,利用RFE算法进行特征筛选,得到一个新的特征子集。接着,利用交叉验证计算新特征子集下的模型得分,并将得分保存在score列表中。最后,通过matplotlib库将score列表中的得分绘制成图表,以便直观地查看得分随特征数量的变化情况。
需要注意的是,代码中还将特征得分排名和每次交叉迭代各个特征得分保存到了csv文件中,并注释了相关代码。
阅读全文
相关推荐
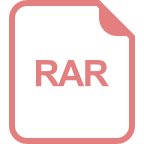
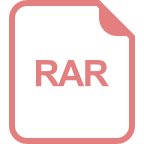
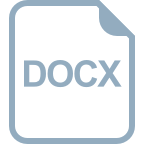













