用pygame输出一个五子棋游戏
时间: 2023-06-16 13:04:36 浏览: 72
好的,下面是一个简单的五子棋游戏的代码,使用 Pygame 库进行绘制和事件处理。
```python
import pygame
from pygame.locals import *
# 初始化 Pygame
pygame.init()
# 设置窗口大小
SCREEN_SIZE = (600, 600)
screen = pygame.display.set_mode(SCREEN_SIZE)
# 设置窗口标题
pygame.display.set_caption('五子棋')
# 定义棋盘格子大小和棋子半径
GRID_SIZE = 40
RADIUS = GRID_SIZE // 2 - 2
# 定义棋盘大小和边距
BOARD_SIZE = 15
MARGIN = 40
# 定义棋子颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
# 定义棋盘二维数组,表示棋子的位置
board = [[0 for x in range(BOARD_SIZE)] for y in range(BOARD_SIZE)]
# 定义当前下棋方,1 表示黑方,-1 表示白方
current_player = 1
def draw_board():
# 绘制棋盘背景
screen.fill((200, 200, 200))
# 绘制棋盘网格
for i in range(BOARD_SIZE):
x = MARGIN + i * GRID_SIZE
pygame.draw.line(screen, BLACK, (x, MARGIN), (x, SCREEN_SIZE[1] - MARGIN))
pygame.draw.line(screen, BLACK, (MARGIN, x), (SCREEN_SIZE[0] - MARGIN, x))
# 绘制棋子
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == 1:
pygame.draw.circle(screen, BLACK, (MARGIN + i * GRID_SIZE, MARGIN + j * GRID_SIZE), RADIUS)
elif board[i][j] == -1:
pygame.draw.circle(screen, WHITE, (MARGIN + i * GRID_SIZE, MARGIN + j * GRID_SIZE), RADIUS)
def get_pos(x, y):
# 将鼠标点击位置转换成棋盘坐标
i = (x - MARGIN) // GRID_SIZE
j = (y - MARGIN) // GRID_SIZE
return i, j
def check_win(i, j):
# 检查是否有五子连成一线,如果有则返回 True,否则返回 False
directions = [(1, 0), (0, 1), (1, 1), (1, -1)]
for dx, dy in directions:
count = 1
x, y = i, j
while count < 5:
x, y = x + dx, y + dy
if x < 0 or x >= BOARD_SIZE or y < 0 or y >= BOARD_SIZE or board[x][y] != current_player:
break
count += 1
x, y = i, j
while count < 5:
x, y = x - dx, y - dy
if x < 0 or x >= BOARD_SIZE or y < 0 or y >= BOARD_SIZE or board[x][y] != current_player:
break
count += 1
if count >= 5:
return True
return False
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == QUIT:
running = False
elif event.type == MOUSEBUTTONDOWN:
# 处理鼠标点击事件
if current_player == 1:
# 黑方下棋
i, j = get_pos(event.pos[0], event.pos[1])
if board[i][j] == 0:
board[i][j] = current_player
if check_win(i, j):
print('黑方胜利')
running = False
current_player = -current_player
else:
# 白方下棋
i, j = get_pos(event.pos[0], event.pos[1])
if board[i][j] == 0:
board[i][j] = current_player
if check_win(i, j):
print('白方胜利')
running = False
current_player = -current_player
# 绘制界面
draw_board()
pygame.display.update()
# 退出 Pygame
pygame.quit()
```
这个代码运行后会显示一个窗口,你可以用鼠标点击棋盘上的空白格子来下棋。黑方先下,如果有五子连成一线则判定胜利。
相关推荐
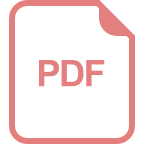
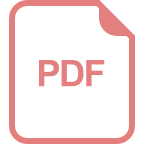














