用pygame写一个五子棋
时间: 2023-10-09 12:10:03 浏览: 47
由于我是AI语言模型,无法直接编写代码,但我可以提供一个简单的五子棋实现思路:
1. 导入pygame模块
2. 定义游戏窗口大小、棋盘格子大小等参数
3. 初始化pygame并设置窗口
4. 绘制棋盘和棋子
5. 定义落子函数,判断是否合法并落子
6. 定义胜利判断函数,判断是否有五子连珠
7. 定义重新开始游戏函数
8. 定义主函数,循环监听事件并执行对应函数
具体实现方法可以参考以下代码:
``` python
import pygame
# 定义窗口大小和格子大小
WINDOW_WIDTH = 600
WINDOW_HEIGHT = 600
GRID_SIZE = 30
# 定义棋盘大小和初始位置
BOARD_SIZE = 15
BOARD_LEFT = (WINDOW_WIDTH - BOARD_SIZE * GRID_SIZE) // 2
BOARD_TOP = (WINDOW_HEIGHT - BOARD_SIZE * GRID_SIZE) // 2
# 定义棋子颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
# 初始化pygame
pygame.init()
# 设置窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption("五子棋")
# 绘制棋盘
def draw_board():
for i in range(BOARD_SIZE):
pygame.draw.line(window, BLACK, (BOARD_LEFT, BOARD_TOP + i * GRID_SIZE), (BOARD_LEFT + (BOARD_SIZE - 1) * GRID_SIZE, BOARD_TOP + i * GRID_SIZE), 1)
pygame.draw.line(window, BLACK, (BOARD_LEFT + i * GRID_SIZE, BOARD_TOP), (BOARD_LEFT + i * GRID_SIZE, BOARD_TOP + (BOARD_SIZE - 1) * GRID_SIZE), 1)
# 绘制棋子
def draw_piece(row, col, color):
pygame.draw.circle(window, color, (BOARD_LEFT + col * GRID_SIZE, BOARD_TOP + row * GRID_SIZE), GRID_SIZE // 2 - 2)
# 落子函数
def drop_piece(row, col, color):
if board[row][col] == 0:
board[row][col] = color
draw_piece(row, col, BLACK if color == 1 else WHITE)
return True
else:
return False
# 胜利判断函数
def check_win(row, col, color):
count = 0
# 水平方向
for c in range(col - 4, col + 5):
if c < 0 or c >= BOARD_SIZE:
continue
if board[row][c] == color:
count += 1
else:
count = 0
if count >= 5:
return True
# 垂直方向
count = 0
for r in range(row - 4, row + 5):
if r < 0 or r >= BOARD_SIZE:
continue
if board[r][col] == color:
count += 1
else:
count = 0
if count >= 5:
return True
# 左上-右下方向
count = 0
for i in range(-4, 5):
r = row + i
c = col + i
if r < 0 or r >= BOARD_SIZE or c < 0 or c >= BOARD_SIZE:
continue
if board[r][c] == color:
count += 1
else:
count = 0
if count >= 5:
return True
# 右上-左下方向
count = 0
for i in range(-4, 5):
r = row + i
c = col - i
if r < 0 or r >= BOARD_SIZE or c < 0 or c >= BOARD_SIZE:
continue
if board[r][c] == color:
count += 1
else:
count = 0
if count >= 5:
return True
return False
# 重新开始游戏函数
def restart_game():
global board
board = [[0] * BOARD_SIZE for _ in range(BOARD_SIZE)]
window.fill(WHITE)
draw_board()
# 主函数
def main():
global board
# 初始化棋盘
board = [[0] * BOARD_SIZE for _ in range(BOARD_SIZE)]
# 绘制棋盘
window.fill(WHITE)
draw_board()
# 游戏循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.MOUSEBUTTONDOWN:
if event.button == 1:
x, y = event.pos
row = (y - BOARD_TOP) // GRID_SIZE
col = (x - BOARD_LEFT) // GRID_SIZE
if row >= 0 and row < BOARD_SIZE and col >= 0 and col < BOARD_SIZE:
if drop_piece(row, col, 1):
if check_win(row, col, 1):
print("黑方胜利!")
restart_game()
else:
# AI落子
pass
else:
print("此处已有棋子,请重新落子!")
# 更新窗口
pygame.display.update()
if __name__ == "__main__":
main()
```
由于是简单的示例代码,其中AI部分没有实现。如果要实现AI部分,可以使用Minimax算法或Alpha-Beta剪枝算法等。
相关推荐
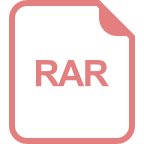
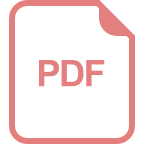














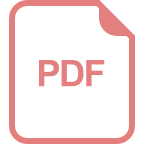