编写一个C语言程序,要求实现带头结点的链表的以下功能: 链表初始化、链表的建立(分别按头插法和尾插法两种方法建立两个链表:链表A和链表B,要求提供机会在键盘上分别依次输入两个链表的元素值)、链表A的按值查找(提供机会在键盘上输入要查找的值)、链表A的遍历、链表A的插入(提供机会在键盘上输入要插入的值和插入的位置)、链表A的删除(提供机会在键盘上输入要删除的值)、最后再把链表A和链表B进行合并,合并之后成链表C,要求链表C仍有序。(要求每一步都给出注释)
时间: 2023-05-27 15:01:50 浏览: 55
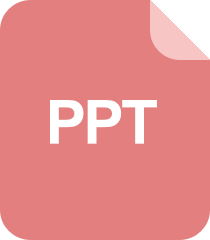
前插入结点算法-关于c语言链表的ppt
```
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int data;
struct ListNode *next;
} ListNode, *ListPtr;
// 初始化链表
void initList(ListPtr *L) {
*L = (ListNode *) malloc(sizeof(ListNode));
(*L)->next = NULL;
}
// 头插法建立链表
void createListHead(ListPtr L) {
int x;
ListNode *p;
printf("Please input elements of the list(end with -1): ");
scanf("%d", &x);
while (x != -1) {
p = (ListNode *) malloc(sizeof(ListNode));
p->data = x;
p->next = L->next;
L->next = p;
scanf("%d", &x);
}
}
// 尾插法建立链表
void createListTail(ListPtr L) {
int x;
ListNode *p, *tail;
tail = L;
printf("Please input elements of the list(end with -1): ");
scanf("%d", &x);
while (x != -1) {
p = (ListNode *) malloc(sizeof(ListNode));
p->data = x;
tail->next = p;
tail = p;
scanf("%d", &x);
}
tail->next = NULL;
}
// 按值查找
int findList(ListPtr L, int x) {
ListNode *p = L->next;
int i = 0;
while (p) {
i++;
if (p->data == x) {
printf("The index of %d in the list is %d.\n", x, i);
return i;
}
p = p->next;
}
printf("%d is not in the list.\n", x);
return 0;
}
// 遍历链表
void printList(ListPtr L) {
ListNode *p = L->next;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
// 插入节点
void insertList(ListPtr L, int x, int n) {
ListNode *p = L, *q;
int i = 0;
while (p && i < n - 1) {
p = p->next;
i++;
}
if (!p || i > n - 1) {
printf("Invalid position to insert.\n");
return;
}
q = (ListNode *) malloc(sizeof(ListNode));
q->data = x;
q->next = p->next;
p->next = q;
printf("Inserted %d into the list at position %d.\n", x, n);
}
// 删除节点
void deleteList(ListPtr L, int x) {
ListNode *p = L->next, *q = L;
while (p) {
if (p->data == x) {
q->next = p->next;
free(p);
printf("Deleted %d from the list.\n", x);
return;
}
q = p;
p = p->next;
}
printf("%d is not in the list.\n", x);
}
// 合并链表
void mergeList(ListPtr La, ListPtr Lb, ListPtr Lc) {
ListNode *pa = La->next, *pb = Lb->next, *pc = Lc;
while (pa && pb) {
if (pa->data <= pb->data) {
pc->next = pa;
pa = pa->next;
} else {
pc->next = pb;
pb = pb->next;
}
pc = pc->next;
}
pc->next = pa ? pa : pb;
free(Lb);
}
int main() {
ListPtr La, Lb, Lc;
int x, n;
// 初始化链表
initList(&La);
initList(&Lb);
initList(&Lc);
// 头插法建立La
createListHead(La);
printList(La);
// 尾插法建立Lb
createListTail(Lb);
printList(Lb);
// 查找节点
printf("Please input the element you want to find: ");
scanf("%d", &x);
findList(La, x);
// 插入节点
printf("Please input the element and position(inserted from head) you want to insert: ");
scanf("%d%d", &x, &n);
insertList(La, x, n);
printList(La);
// 删除节点
printf("Please input the element you want to delete: ");
scanf("%d", &x);
deleteList(La, x);
printList(La);
// 合并链表
mergeList(La, Lb, Lc);
printList(Lc);
return 0;
}
```
阅读全文
相关推荐
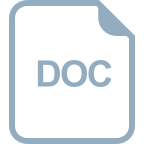
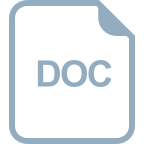















