player.playNext = function playNext(){ if(this._songlist.length===0)return; var index = this.getSongListIndex(player._currentSong); switch (current_play_mod) { case "list": /**列表循环*/ if(index === (this._songlist.length-1)){ this.playSong(this._songlist[0]); } else { this.playSong(this._songlist[index+1]); } break; case "order":/**顺序播放*/ if(index < (this._songlist.length-1)){ this.playSong(this._songlist[index+1]); } break; case "random":/**随机播放*/ this.playSong(this._songlist[Math.floor(Math.random()*this._songlist.length)]); break; case "single":/**单曲循环*/ this.playSong(this._songlist[index]); break; } 解释
时间: 2024-01-28 14:05:45 浏览: 101
这是一段 JavaScript 代码,它定义了一个名为 `playNext` 的函数。该函数的作用是根据当前的播放模式,选择下一首歌曲进行播放。这个函数依赖于一个名为 `_songlist` 的数组,该数组包含了所有要播放的歌曲,并且依赖于一个名为 `_currentSong` 的变量,表示当前正在播放的歌曲。
该函数首先检查 `_songlist` 数组的长度,如果长度为 0,则直接返回,因为没有可播放的歌曲。否则,它会调用 `getSongListIndex` 函数来获取当前播放歌曲在 `_songlist` 数组中的索引。
接下来,根据当前的播放模式进行判断。如果当前播放模式为列表循环,则判断当前播放的歌曲是否为列表中的最后一首,如果是,则播放列表中的第一首歌曲,否则播放下一首歌曲。如果当前播放模式为顺序播放,则判断当前播放的歌曲是否为列表中的最后一首,如果不是,则播放下一首歌曲。如果当前播放模式为随机播放,则随机选择一首歌曲进行播放。如果当前播放模式为单曲循环,则重复播放当前歌曲。
最后,根据选择的歌曲,调用 `playSong` 函数来播放该歌曲。
相关问题
playAudio: function() { // 创建内部音频上下文 const audioContext = wx.createInnerAudioContext(); // 设置音频链接 audioContext.src = 'https://song.gushiwen.cn/song/jiangweiwei/870828ca8aaa.mp3'; // 播放音频 audioContext.play(); },
你的代码已经实现了播放音频的功能。通过`wx.createInnerAudioContext()`方法创建了内部音频上下文对象`audioContext`,并设置了音频链接`audioContext.src`。然后,调用`audioContext.play()`方法来播放音频。
这段代码可以在微信小程序中使用,当调用`playAudio()`函数时,会创建内部音频上下文对象并设置音频链接,然后开始播放音频。
如果你希望实现点击上一首和下一首的功能,你需要对代码进行一些修改。你可以将音频链接和操作相关的逻辑提取到一个独立的方法中,并在点击上一首和下一首按钮时调用该方法。以下是一个修改后的示例代码:
```javascript
Page({
data: {
audioList: [
'https://song.gushiwen.cn/song/jiangweiwei/870828ca8aaa.mp3',
'音频链接2',
'音频链接3'
],
currentIndex: 0,
audioContext: null
},
onLoad() {
// 创建内部音频上下文
this.data.audioContext = wx.createInnerAudioContext();
// 设置初始音频链接
this.data.audioContext.src = this.data.audioList[this.data.currentIndex];
},
// 上一首按钮点击事件
playPrev() {
let index = this.data.currentIndex - 1;
if (index < 0) {
index = this.data.audioList.length - 1;
}
this.setData({
currentIndex: index
});
this.updateAudio();
},
// 下一首按钮点击事件
playNext() {
let index = this.data.currentIndex + 1;
if (index >= this.data.audioList.length) {
index = 0;
}
this.setData({
currentIndex: index
});
this.updateAudio();
},
// 播放/暂停按钮点击事件
togglePlay() {
if (this.data.audioContext.paused) {
this.data.audioContext.play();
} else {
this.data.audioContext.pause();
}
},
// 更新音频链接并播放
updateAudio() {
this.data.audioContext.stop();
this.data.audioContext.src = this.data.audioList[this.data.currentIndex];
this.data.audioContext.play();
}
});
```
在这个修改后的代码中,我们在`onLoad`生命周期函数中创建了内部音频上下文对象`audioContext`,并设置了初始音频链接。然后,在`playPrev`和`playNext`方法中,我们更新了`currentIndex`的值,并调用了`updateAudio`方法来更新音频链接并播放。
这样,你就可以实现点击上一首和下一首按钮时切换音频并自动播放的功能了。同时,播放/暂停按钮可以控制音频的播放和暂停状态。记得将示例代码中的"音频链接2"和"音频链接3"替换为实际的音频链接。
<view class="container"> <view class="playlist"> <view class="playlist-header">播放列表</view> <scroll-view class="playlist-content" scroll-y="true"> <view wx:for="{{playList}}" wx:key="index" class="playlist-item" bindtap="playMusic" data-index="{{index}}"> {{item.name}} </view> </scroll-view> </view> <audio id="audio" binderror="audioError" bindtimeupdate="updateTime" bindended="playNext"></audio> <view class="player"> <view class="player-info"> <view class="player-name">{{currentMusic.name}}</view> <view class="player-time">{{formatTime(currentTime)}} / {{formatTime(duration)}}</view> </view> <view class="player-control"> <button class="player-btn" bindtap="togglePlay"> <image src="{{isPlaying ? 'pause.png' : 'play.png'}}"></image> </button> <button class="player-btn" bindtap="playPrev"> <image src="prev.png"></image> </button> <button class="player-btn" bindtap="playNext"> <image src="next.png"></image> </button> </view> </view></view>的样式部分
.container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
background-color: #f5f5f5;
}
.playlist {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
background-color: #fff;
padding: 20px;
border-radius: 5px;
box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.1);
margin-bottom: 20px;
}
.playlist-header {
font-size: 20px;
font-weight: bold;
margin-bottom: 10px;
}
.playlist-content {
height: 150px;
width: 100%;
margin-bottom: 10px;
}
.playlist-item {
display: flex;
align-items: center;
justify-content: center;
height: 30px;
font-size: 16px;
cursor: pointer;
}
.player {
display: flex;
align-items: center;
justify-content: center;
background-color: #fff;
padding: 20px;
border-radius: 5px;
box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.1);
}
.player-info {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
margin-right: 20px;
}
.player-name {
font-size: 24px;
font-weight: bold;
margin-bottom: 10px;
}
.player-time {
font-size: 14px;
color: #666;
}
.player-control {
display: flex;
align-items: center;
justify-content: center;
}
.player-btn {
background-color: transparent;
border: none;
margin-right: 10px;
cursor: pointer;
}
.player-btn:last-child {
margin-right: 0;
}
.player-btn:focus {
outline: none;
}
.player-btn:active {
transform: scale(0.9);
}
.image {
height: 20px;
width: 20px;
}
阅读全文
相关推荐
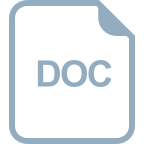
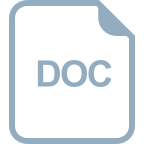
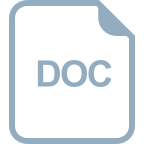
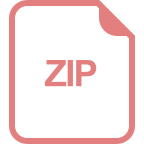
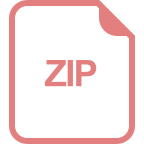
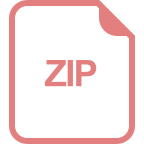
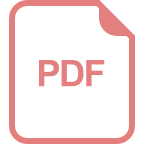
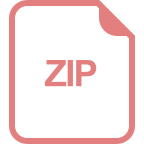
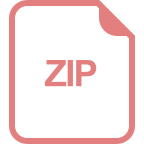


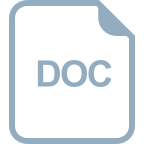
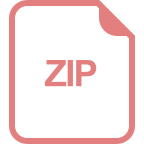