ValueError: Input 0 of layer sequential is incompatible with the layer: expected axis -1 of input shape to have value 6 but received input with shape (None, 31)
时间: 2024-04-10 17:28:09 浏览: 133
这个错误是由于输入数据的维度不匹配导致的。在这个情况下,你正在使用一个包含多个层的 Sequential 模型,其中第一个层期望输入的最后一个维度(axis -1)具有值 6,但实际输入的维度是 (None, 31)。
要解决这个问题,你可以检查输入数据的维度是否与模型期望的维度匹配。如果你知道输入数据应该具有 6 个特征,你可以使用 reshape() 函数调整输入数据的形状,使其最后一个维度为 6。例如:
```python
import numpy as np
# 假设你的输入数据是 input_data
input_data = np.random.rand(100, 31) # 生成一个随机的输入数据
# 调整输入数据的形状
input_data_reshaped = input_data.reshape((100, 6))
# 然后将调整后的输入数据传递给模型进行训练或预测
model.fit(input_data_reshaped, ...)
```
另外,你还可以检查模型的定义,确保模型中的层与输入数据的维度匹配。
相关问题
ValueError: Input 0 of layer sequential is incompatible with the layer: expected ndim=3, found ndim=2. Full shape received: (None, 10)怎么修改代码
这个错误通常是由于输入数据维度不匹配导致的。你可以检查一下输入数据的维度是否正确。如果输入数据的维度是 `(batch_size, sequence_length)`,而模型期望的输入维度应该是 `(batch_size, sequence_length, embedding_size)`,则会出现这个错误。
你可以在模型的第一层添加一个 `Input` 层,并指定正确的 `input_shape` 参数。例如,如果你的输入数据维度是 `(batch_size, sequence_length)`,而嵌入层的维度是 `embedding_size`,则可以这样定义输入层:
```
from tensorflow.keras.layers import Input, Embedding, LSTM, Dense
from tensorflow.keras.models import Model
input_layer = Input(shape=(sequence_length,))
embedding_layer = Embedding(input_dim=vocab_size, output_dim=embedding_size)(input_layer)
lstm_layer = LSTM(units=hidden_size)(embedding_layer)
output_layer = Dense(units=output_size, activation='softmax')(lstm_layer)
model = Model(inputs=input_layer, outputs=output_layer)
```
在这个例子中,我们首先定义了一个输入层 `input_layer`,并指定了 `input_shape=(sequence_length,)`。然后我们添加了一个嵌入层 `embedding_layer`,它的输入是 `input_layer` 的输出,输出维度是 `embedding_size`。接下来我们添加了一个 LSTM 层 `lstm_layer`,它的输入是 `embedding_layer` 的输出,输出维度是 `hidden_size`。最后我们添加了一个输出层 `output_layer`,它的输入是 `lstm_layer` 的输出,输出维度是 `output_size`,激活函数是 `softmax`。
这样定义模型之后,你可以使用 `model.fit()` 方法来训练模型。在训练之前,你需要将输入数据转换成正确的维度,例如,如果你有一个形状为 `(batch_size, sequence_length)` 的输入数据张量 `x`,你可以这样将它转换成 `(batch_size, sequence_length, embedding_size)` 的张量:
```
x = np.random.randint(0, vocab_size, size=(batch_size, sequence_length))
x = embedding_layer(x)
```
ValueError: Input 0 of layer dense is incompatible with the layer: : expected min_ndim=2, found ndim=0. Full shape received: []
这个错误通常是因为您传递给 `tf.keras.layers.Dense` 函数的输入张量是一个标量,而密集层需要一个至少是二维的输入张量。
请检查您的代码,确保您传递给 `tf.keras.layers.Dense` 函数的张量维度正确。如果您的输入张量是一个标量,则需要将其转换为一个至少是二维的张量,例如:
```
inputs = tf.keras.Input(shape=(1,))
Q = tf.keras.layers.Dense(units, activation=tf.nn.relu)(inputs)
```
这里,我们将标量张量的形状指定为 `(1,)`,以将其转换为一个二维张量。这样,您就可以避免上述错误,并确保您的代码可以正常运行。
阅读全文
相关推荐
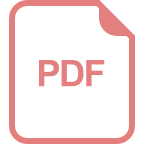











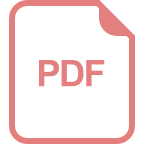
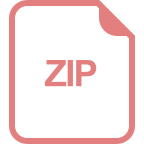
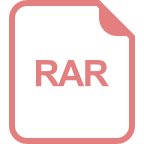
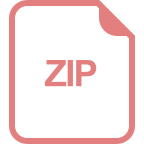